Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial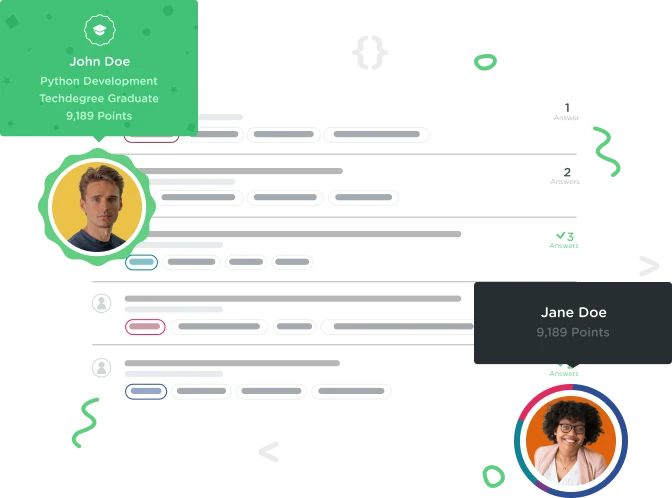
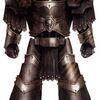
Cole Smith
Web Development Techdegree Student 13,802 Pointshow do I properly return this new array?
need help with this challenge. I've gotten this far but I definitely know something isn't quite right with my return statement, do I need to use [] at all there?
const years = [1989, 2015, 2000, 1999, 2013, 1973, 2012];
let century20;
// century20 should be: [1989, 2000, 1999, 1973]
// Write your code below
century20 = years.filter (year => {
if (year.index <= 2000) {
return century20[year];
}
});
console.log(century20);
1 Answer

Emmanuel C
10,636 PointsHey Cole,
So, filter takes a function as a parameter that is applied to each item in the array. When you create the arrow function with year as a parameter. year is the item in the years array. So doing year.index would be like doing 1989.index, in the first iteration.
Also, what returns from that inner function should be a boolean(true/false) as to whether that year item passes the condition it's supposed to be filtered by. Basically, just checking and returning if the year <= 2000 is what that inner function is supposed to do. Something like this...
century20 = years.filter(year => { return (year <= 2000) });
You can shorten is further with arrow functions by removing the curly braces, the return, and the parenthesis too if you'd like. Like...
century20 = years.filter(year => year <= 2000);
Cole Smith
Web Development Techdegree Student 13,802 PointsCole Smith
Web Development Techdegree Student 13,802 Pointsalright this makes sense now, my code was saying to iterate down from 2000 not the whole list. thanks for the help!