Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial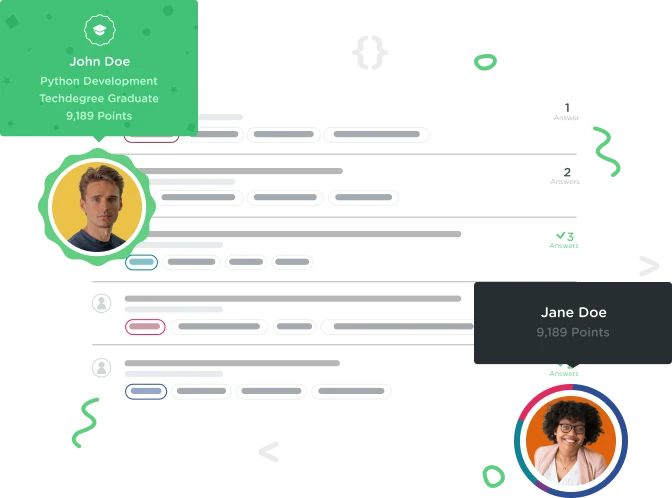

Chuck Anderson
3,278 PointsHow do i put a variable into a raw string?
I need to find all the words, with a given length (num) or longer, in a string (str) using a regex. I can get it work with a hard coded number like this re.findall(r'\w{4,}', str)
but how can I use a variable so i can have different lengths?
I've tried this re.findall(r'\w{num,}', str)
and re.findall(r'\w{%num,}', str)
but I get an empty list back.
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(c, s):
return re.findall(r'\w{c,}', s)
5 Answers
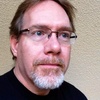
Chris Freeman
Treehouse Moderator 68,423 PointsOther ways to embed variable values into raw strings:
>>> import re
>>> c = 5
>>> s = "this and that alrighty to booth"
>>> re.findall(r'\w{' + str(c) + ',}', s)
['alrighty', 'booth']
>>> re.findall(r'\w{%s,}' % c, s)
['alrighty', 'booth']
>>> re.findall(r'\w{{{},}}'.format(c), s)
['alrighty', 'booth']
>>> re.findall(r'\w{{{c},}}'.format(c=c), s)
['alrighty', 'booth']
>>> re.findall(fr'\w{{{c},}}', s)
['alrighty', 'booth']
>>>
Post back if you need more help. Good luck!!
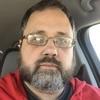
Mark Sebeck
Treehouse Moderator 37,776 PointsI'm sure there is a better way to do this but this did pass the challenge.
return re.findall(r'\w{' + str(c) + ',}', s)
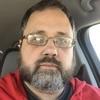
Mark Sebeck
Treehouse Moderator 37,776 PointsI'm actually going to be retaking this course soon because I am reviewing Python. I'll let you know if I find something better.

Chuck Anderson
3,278 PointsOK, thank you for the response! i didn't realize i could cast a string inside the raw string.
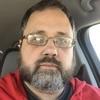
Mark Sebeck
Treehouse Moderator 37,776 PointsYou're welcome. If you find a cleaner way let me know. Even though it works I'm not sure it's the best way.

jaesonshin
Python Development Techdegree Graduate 9,939 PointsThanks, Mark and Chris!
re.findall(r'\w{{{},}}'.format(c), s)
so, for the code above, out of the three sets of {}, the second set is to escape the innermost one(which is for the '.format method) and the comma, right?
ok, got it!
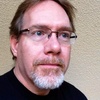
Chris Freeman
Treehouse Moderator 68,423 Pointsre.findall(r'\w{{{},}}'.format(c), s)
xxff xx
# x marks escaped pair that will be replaced
# by single curly bracket for use in regex
# f marks curly bracket used by format that
# will be replaced by substitution

jaesonshin
Python Development Techdegree Graduate 9,939 PointsThanks!! got it!!!
Mark Sebeck
Treehouse Moderator 37,776 PointsMark Sebeck
Treehouse Moderator 37,776 PointsThanks Chris! I knew there had to be a cleaner way
jaesonshin
Python Development Techdegree Graduate 9,939 Pointsjaesonshin
Python Development Techdegree Graduate 9,939 PointsHi Chris,
I have two questions about the code that you posted.
Why do we need + for the code below?
re.findall(r'\w{' + str(c) + ',}', s)
Why do we need three sets of curly braces instead of two for the code below?
re.findall(r'\w{{{},}}'.format(c), s)
Mark Sebeck
Treehouse Moderator 37,776 PointsMark Sebeck
Treehouse Moderator 37,776 PointsHopefully Chris doesn't mind me jumping in here. I retook the regular expression class and have studied Chris's solutions after the last time I answered this question.
On number 1 the '+' are because we are simply concatenating a string. Just like "My name is " + name + "."
Number 2 is more complicated. So for the findall we want
re.findall(r'\w{c,}', s)
Where the c will be replaced with our variable using format.
To get those brackets we need to double the brackets because that's how you escape them to insert them into a string. But then we want to replace the c with whats in the format string so we need another set of brackets replacing the c.
Hope that clears it up.
Feel free to jump in Chris if you can explain it clearer.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThanks Mark Sebeck! I answered a similar question yesterday regarding the concatenation.
First, look at the whole regex:
The first and last are raw strings. The second creates a string from the variable
count
. Using the + concatenates the three strings into one string.Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 Points