Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial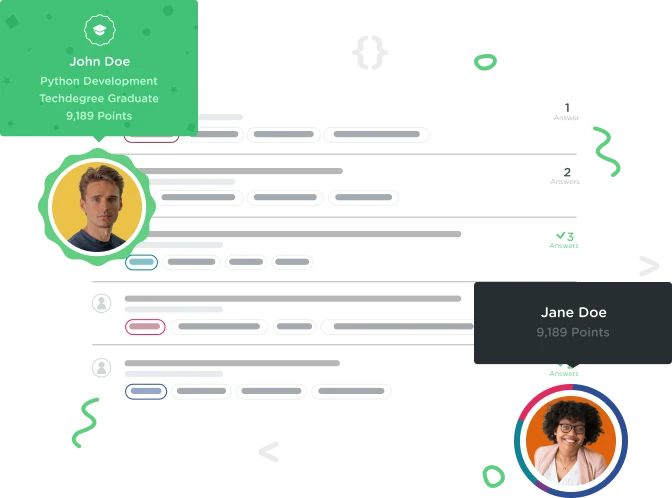
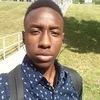
William Thompson
4,580 PointsHow do I put content in a table view that has already been defined in FXML. 1, FXML. 2, CONTROLLER, 3, MAIN 4,PRODUCT
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?> <?import javafx.scene.control.ButtonBar?> <?import javafx.scene.control.Label?> <?import javafx.scene.control.SplitPane?> <?import javafx.scene.control.TableColumn?> <?import javafx.scene.control.TableView?> <?import javafx.scene.control.TextArea?> <?import javafx.scene.control.TextField?> <?import javafx.scene.control.ToolBar?> <?import javafx.scene.layout.AnchorPane?> <?import javafx.scene.layout.ColumnConstraints?> <?import javafx.scene.layout.GridPane?> <?import javafx.scene.layout.RowConstraints?> <?import javafx.scene.text.Font?> <?import javafx.scene.text.Text?>
<GridPane alignment="center" hgap="10" vgap="10" xmlns="http://javafx.com/javafx/8.0.112" xmlns:fx="http://javafx.com/fxml/1" fx:controller="sample.Controller"> <columnConstraints> <ColumnConstraints /> </columnConstraints> <rowConstraints> <RowConstraints /> <RowConstraints /> </rowConstraints> <children> <SplitPane dividerPositions="0.29797979797979796" prefHeight="500.0" prefWidth="1000.00" GridPane.rowIndex="1"> <items> <AnchorPane minHeight="0.0" minWidth="0.0" prefHeight="160.0" prefWidth="100.0"> <children> <Label layoutX="14.0" layoutY="45.0" text="Item Name" /> <TextField id="nameBox" layoutX="129.0" layoutY="40.0" fx:id="myNameField" /> <Label layoutX="14.0" layoutY="92.0" text="Item ID" /> <TextField id="idBox" layoutX="129.0" layoutY="87.0" fx:id="myIdField"/> <TextField id="priceBox" layoutX="129.0" layoutY="137.0" fx:id="myPriceField"/> <Label layoutX="14.0" layoutY="142.0" text="Item Price" /> <TextField id="quantityBox" layoutX="129.0" layoutY="182.0" fx:id="myQuantityField"/> <Label layoutX="14.0" layoutY="187.0" text="Item Quantity" /> <TextField id="locationBox" layoutX="129.0" layoutY="224.0" fx:id="myLocationField"/> <Label layoutX="14.0" layoutY="229.0" text="Item Location" /> <TextArea id="notesBox" layoutX="92.0" layoutY="284.0" prefHeight="200.0" prefWidth="200.0" promptText="Handle with care..." fx:id="myNotesField"/> <Label layoutX="14.0" layoutY="277.0" text="Item Notes" /> <Button layoutX="8.0" layoutY="459.0" mnemonicParsing="false" onAction="#addButtonClicked" text="Add Item" /> </children></AnchorPane> <AnchorPane minHeight="0.0" minWidth="0.0" prefHeight="160.0" prefWidth="100.0"> <children> <TableView id="inventoryTable" layoutX="-1.0" prefHeight="498.0" prefWidth="697.0" fx:id="myInventoryTable"> <columns> <TableColumn id="nameCol" prefWidth="114.0" text="Name" fx:id="nameCol" /> <TableColumn id="idCol" prefWidth="63.0" text="ID" fx:id="idCol"/> <TableColumn id="priceCol" prefWidth="69.0" text="Price" fx:id="priceCol"/> <TableColumn id="quantityCol" prefWidth="65.0" text="Quantity" fx:id="quantityCol"/> <TableColumn id="locationCol" prefWidth="113.0" text="Location" fx:id="locationCol"/> <TableColumn prefWidth="272.0" text="Notes" fx:id="notesCol"/> </columns> </TableView> </children></AnchorPane> </items> </SplitPane> <ToolBar prefHeight="40.0" prefWidth="200.0"> <items> <Label text="Inventory Management System" textFill="#07b226"> <font> <Font size="19.0" /> </font></Label> <ButtonBar prefHeight="40.0" prefWidth="200.0"> <buttons> <Text fill="#f50e0e" strokeType="OUTSIDE" strokeWidth="0.0" text="611"> <font> <Font name="Raleway" size="21.0" /> </font> </Text> </buttons></ButtonBar> <Text fill="#e80909" strokeType="OUTSIDE" strokeWidth="0.0" text="Solutions"> <font> <Font name="Raleway" size="21.0" /> </font> </Text> <ButtonBar prefHeight="40.0" prefWidth="200.0"> <buttons> <Button mnemonicParsing="false" text="New File" textFill="#d01c1c" /> <Button mnemonicParsing="false" text="Edit File" textFill="#d01c1c" /> <Button mnemonicParsing="false" text="Open File" textFill="#d01c1c" /> <Button mnemonicParsing="false" text="Info" textFill="#d01c1c" /> </buttons> </ButtonBar> </items> </ToolBar> </children> </GridPane>
import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.scene.control.*; import javafx.scene.control.cell.PropertyValueFactory;
public class Controller{
// getting the fields from the FXML and connecting them using their fxid
@FXML private TextField myNameField;
@FXML private TextField myIdField;
@FXML private TextField myPriceField;
@FXML private TextField myQuantityField;
@FXML private TextField myLocationField;
@FXML private TextArea myNotesField;
// getting the table view from the FXML and connecting it using its fxid. It also connects the table view with the product class
@FXML TableView <Product> myInventoryTable;
public void setMyInventoryTable(TableView<Product> myInventoryTable) {
this.myInventoryTable = myInventoryTable;
}
@FXML private TableColumn <Product, String> nameCol = new TableColumn<>("Name");
@FXML private TableColumn<Product, String> idCol = new TableColumn<>("ID");
@FXML private TableColumn <Product, String>priceCol = new TableColumn<>("Price");
@FXML private TableColumn <Product, String>quantityCol = new TableColumn<>("Quantity");
@FXML private TableColumn <Product, String>locationCol = new TableColumn<>("Location");
@FXML private TableColumn <Product, String>notesCol = new TableColumn<>("Notes");
public void addButtonClicked(){
System.out.println("it worked");
System.out.println(myNameField.getText().toString());
System.out.println(myIdField.getText().toString());
System.out.println(myPriceField.getText().toString());
System.out.println(myQuantityField.getText().toString());
System.out.println(myLocationField.getText().toString());
System.out.println(myNotesField.getText().toString());
}
// get all of the products
public ObservableList<Product> getProduct(){
ObservableList<Product> products = FXCollections.observableArrayList();
products.add(new Product("laptop", "001", 800.00, 5, "store 1", "be careful"));
products.add(new Product("processor", "002", 850.00, 5, "store 1", "be careful"));
products.add(new Product("monitor", "003", 600.00, 5, "store 1", "be careful"));
products.add(new Product("ram", "004", 500.00, 5, "store 1", "be careful"));
products.add(new Product("power supply", "005", 250.00, 5, "store 1", "be careful"));
return products;
}
}
import com.sun.org.apache.xpath.internal.functions.FuncFalse; import javafx.application.Application; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.control.TextArea; import javafx.scene.control.TextField; import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
primaryStage.setTitle("611 Inventory Management System");
primaryStage.setResizable(false);
primaryStage.setScene(new Scene(root, 1000, 600));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
import javafx.fxml.FXML;
/**
-
Created by six11 on 2/7/17. */ public class Product { //member variabes private String name, location, id, notes; private double price; private int quantity;
//1st constructor public Product(){
this.name = ""; this.location = ""; this.notes = ""; this.id = ""; this.price = 0; this.quantity = 0;
}
//2nd constructor public Product(String name, String id, double price, int quantity, String location, String notes){
this.name = name; this.location = location; this.notes = notes; this.id = id; this.price = price; this.quantity = quantity;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}