Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial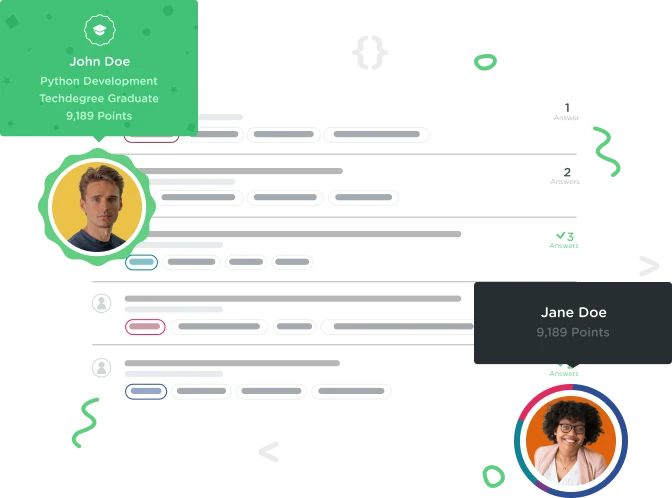

GIFT AKARIS
4,383 Pointshow do I read from multiple files and write to another file.
how do I read from multiple files and write to another file. say
read abc.txt, dfg.txt , ztm.csv and write to klm.txt ?
2 Answers
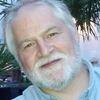
Jeff Muday
Treehouse Moderator 28,717 PointsHow to read from files abc.txt, dfg.txt , ztm.csv and write to klm.txt ?
This is easy as long as you aren't too picky about the output format and the files aren't huge.
# here is a simple minded way to do this.
# read in file1 to data1
read_file1 = open("abc.txt",'r')
data1 = read_file1.read()
read_file1.close()
# read in file2 to data2
read_file2 = open("dfg.txt",'r')
data2 = read_file2.read()
read_file2.close()
# read in file3 to data3
read_file3 = open("ztm.csv",'r')
data3 = read_file3.read()
read_file3.close()
# write operations. make sure we use a newline to keep the data separated
write_file = open('klm.txt','w')
write_file.write(data1 + '\n')
write_file.write(data2 + '\n')
write_file.write(data3 + '\n')
write_file.close()
Here we can get slightly fancier with a list of files to concatenate to the write_file_name. Also note the 'with' statement which is the preferred way to write files since each file is automatically closed once it goes out of scope.
read_file_names = ["abc.txt", "dfg.txt", "ztm.csv"]
write_file_name = 'klm.txt'
for filename in read_file_names:
with open(filename, 'r') as this_file:
# read the entire file into a data buffer
data = this_file.read()
with open(write_file_name, 'a') as that_file:
# append the data buffer to that_file
that_file.write(data + '\n')

Ian Bonyun
4,645 PointsIt's actually very simple: You open each file with the built-in open
function and assign it to a variable. Yup, that simple.
# open all the files
abc_file = open('abc.txt', 'r')
dfg_file = open('dfg.txt', 'r')
ztm_file = open('ztm.csv', 'r')
klm_file = open('klm.txt', 'w')
# do stuff
...
# close all the files
abc_file.close()
def_file.close()
ztm_file.close()
klm.file.close()
Files you want to write to get 'w' as the 2nd argument. Files you want to read from get 'r' as the 2nd argument. Reading is the default so the 'r' can actually be omitted.
Make sure you remember to close all of the files once you're finished with them.
You can also do this with nested with
statements.
with open('abc.txt', 'r') as abc_file:
with open('dfg.txt', 'r') as dfg_file:
... # etc
The with
statements mean they will automatically close when you leave that block. No need to remember to close them yourself. Keep in mind the files will be closed in the reverse order that they're opened.

GIFT AKARIS
4,383 PointsHi Ian,
Thank you for your help
GIFT AKARIS
4,383 PointsGIFT AKARIS
4,383 PointsThanks Jeff,
The second option is exactly what I was after.