Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial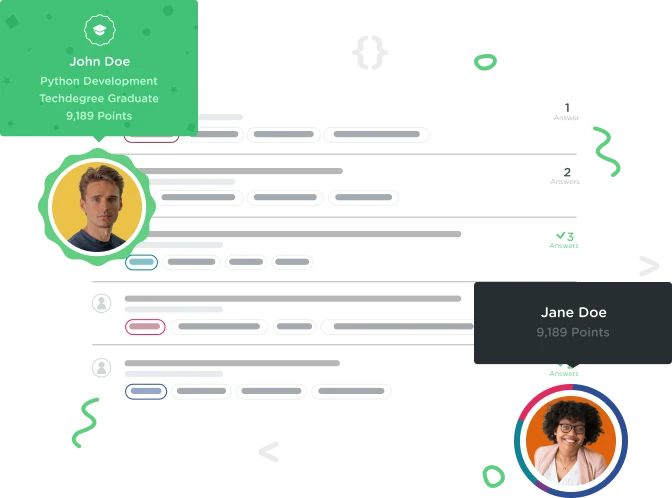

Gary Lehman
2,387 PointsHow do I redefine a class attribute inside of a method?
I'm working through a code challenge question that is as follows:
"Create a method called eat. It should only take self as an argument. Inside of the method, set the is_hungry attribute to False, since the Panda will no longer be hungry when it eats. Also, return a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribute."
I'm confident I have the return string formatted correctly, but I don't understand how to define the name & food within this method & class so that the placeholders in the string can accurately reference them.
I'm also confused as to why I'm receiving the error that the name "name" is not defined; if I can redefine the "is hungry" attribute within this method, why can't I use the same syntax to define self.name = "Bao Bao" within this method?
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
self.name = "Bao Bao"
food = "bamboo"
return f"{name} eats {food}."
2 Answers
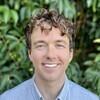
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHi Jack!
"I'm confident I have the return string formatted correctly, but I don't understand how to define the name & food within this method & class so that the placeholders in the string can accurately reference them."
Good question! I'll break it down like this:
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
#the above line says: for all instances of the Panda class, the food attribute is 'bamboo'
Now for the name:
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
#the above line says: for all instances of the Panda class, the food attribute is 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
#the above line sets the "name" attribute on any instance of your Panda class to whatever you set it to.
self.age = age
def eat(self):
self.is_hungry = False
return f'{self.name} eats {self.food}.'
When you click "Check Work," the checker for this Treehouse challenge is running code like this:
ashers_instance = Panda('Bao Bao', 7)
#this creates the instance of the class.
#the __init__ method wants arguments for the name and age attributes, so they get added here.
ashers_instance.eat()
#now it runs the 'eat' method on this instance of your Panda class, which I named ashers_instance
Running ashers_instance.eat() will return "Bao Bao eats bamboo."
'Bao Bao' is the name, since the checker passed in the argument 'Bao Bao' for the name attribute. The name attribute is set when you create an instance. That's why the init method takes name as an argument.
While you can define the "is_hungry" attribute within the eat method, the name attribute it set when you create an instance of the class. That's why you can't define self.name within that method- Python is looking to the dunder init method for the name attribute.
'Bamboo' is the food attribute, because 'bamboo' is the food attribute for ANY instance of the class.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
Your eat method is getting the information about its attributes (self.name, self.age, self.species, etc) from 2 places:
A) The Class attributes
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
If an attribute should always be the same, you make it a Class attribute.
B) The dunder init method.
def __init__(self, name, age):
These are for attributes that might change, depending on the instance. For example:
bao_bao = Panda('Bao Bao', 7)
basi = Panda("Basi", 2)
bobo = Panda("Bobo", 5)
I hope this helps. Let me know if you have further questions!
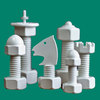
Steven Parker
231,275 PointsWhile "name" is not defined, "self.name" is. Be sure to include the "self." prefix when creating the placeholders in your format string.
Also, the only attribute that should be changed by the new method is "is_hungry". The name and food that were defined when the instance was created should be reported, but not be changed. The info shown in the instructions is for example only.