Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial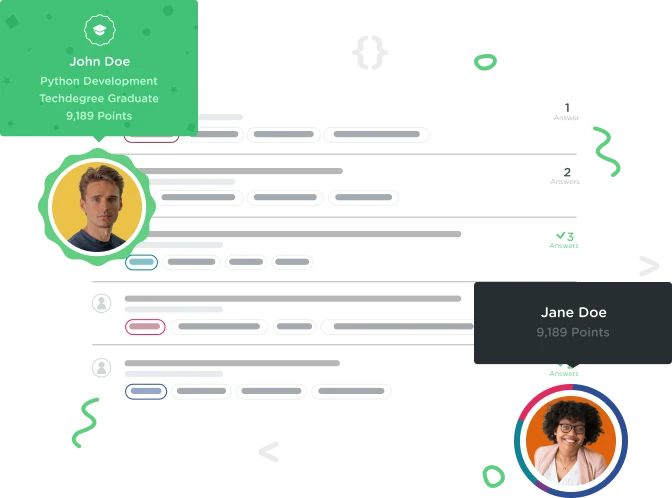

drstrangequark
8,273 PointsHow do I reference the previous value in an array?
The challenge asks me to return true if any value in the array is the same as the preceding value. However, I've looked online and can't find out how to reference the immediately preceding value. I know the code I have written is incorrect but I'm not sure what to do here.
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
if(sequence[this] = sequence[this - 1])
{
return true;
}
}
}
}
2 Answers
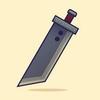
Allan Clark
10,810 PointsI would use a for loop, and just out of preference i would check it the other way around (if the index is the same as the next index). The loop can look something like this:
for(int idx = 0; i < sequence.Length - 1; i++){ //I do Length - 1 here to avoid off by one error, because the last item has
if(sequence[idx] == sequence[idx + 1]) //nothing to compare to.
return true;
}
and if it gets all the way through the loop that means there was no match so just return false.

drstrangequark
8,273 PointsYup, that did it. Thanks guys.
drstrangequark
8,273 Pointsdrstrangequark
8,273 PointsI gave the for loop a try and it returned this error:
RepeatDetector.cs(5,30): error CS0161: `Treehouse.CodeChallenges.RepeatDetector.Scan(int[])': not all code paths return a value Compilation failed: 1 error(s), 0 warnings
Allan Clark
10,810 PointsAllan Clark
10,810 PointsYou need to return something when the for loop completes without hitting the return. So just add this after the loop.
return false;
Alexander La Bianca
15,959 PointsAlexander La Bianca
15,959 PointsYou could modify it a bit to not get that error by doing:
That way all code paths lead to a return value of true or false