Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial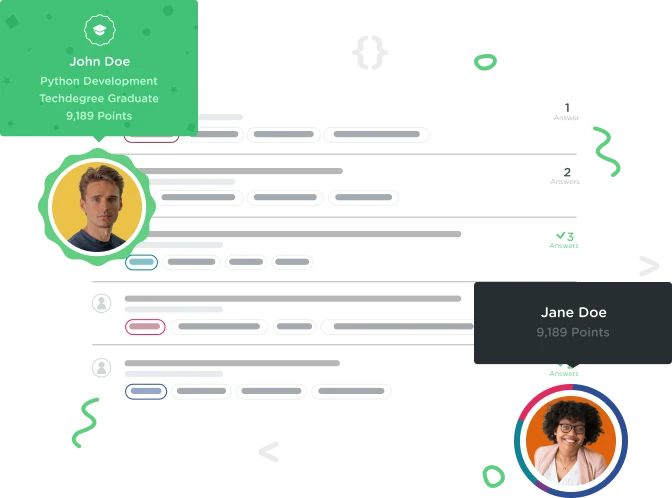
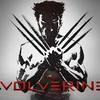
Wolverine .py
2,513 PointsHow do i remove items from my list using del and or/ .remove?
Use .remove() and/or del to remove the string, boolean, and list members of the_list.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.pop(3)
the_list.insert(0, 1)
the_list.remove("a")
del the_list
Lisa Sparks
3,187 Pointsremove.(1)put the item in ()
12 Answers
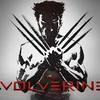
Wolverine .py
2,513 Pointsthank you Tatiana

Tatiana Jamison
6,188 PointsI advanced past this challenge by removing based on value or index. It's not very algorithmic, though. I tried a for loop that tested the item type and wasn't successful initially, but I just tried running this from the console:
for item in the_list:
if type(item) is not int:
the_list.remove(item)
For some reason, the first time through removes every non-int item except the [1,2,3] list object; running it the second time removes the list object. Let us know how you solve it! If anyone has any ideas on why this doesn't remove the list object on the first pass but does on the second pass, let me know.
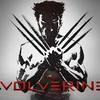
Wolverine .py
2,513 Pointsthe_list.remove([1,2,3])

Jullien Selby
16,320 PointsHere is how I solved it using del.
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.pop(3)
messy_list.insert(0, 1)
messy_list.remove("a")
del messy_list[-2:]
print messy_list ```
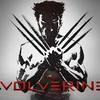
Wolverine .py
2,513 Pointsthanks it worked, i used the_list.remove[1,2,3] i don't know why del didn't work though? any specific reason?

Tatiana Jamison
6,188 PointsDid you use the index of the item in the list? Remember, remove works based on value (so -- .remove("a")), whereas del works based on index in the list (so -- del the_list[0]). If you share your code, it's easier to troubleshoot.
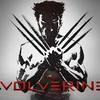
Wolverine .py
2,513 Pointsyes i used the index but its not working

Tatiana Jamison
6,188 PointsHmm. I'd suggest playing around with it in the console, since that seems to provide better error messages than the code challenge. When you use it, ask:
Do you get a syntax error? Are you able to remove another item from a list? (Or an item from another list?) Are you using the correct index? Remember, Python starts its index at 0. Does it remove some other item from the list instead? (This probably means that you have the wrong index.) Remember, you can also use the .index function in Python to find the index. After you remove an item, that will change the index of other items in the list...
Hope that helps!
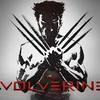
Wolverine .py
2,513 PointsYup nice i got it !! you are awesome thanx i was messing up the index. Thanx again
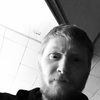
Kody Dibble
2,554 PointsSooo, I'm trying to...
the_list.remove("a") the_list.remove(1,2,3)
does remove not work for int's?
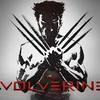
Wolverine .py
2,513 PointsHi Kody, i think it does
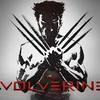
Wolverine .py
2,513 PointsHi Kody, i think it does

Blake Handson
1,642 PointsThis works. All the best.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
var1 = the_list.pop(3) the_list.insert(0, var1)
the_list.remove("a") the_list.remove(False) the_list.remove([1,2,3])

Bakthyar Syed
Courses Plus Student 884 Pointsmessy_list.insert(0,messy_list.pop(3)) for item in messy_list: if type(item) is not int: messy_list.remove(item) messy_list.pop(-1)
print(messy_list)

f4u57
6,743 Pointsmessy_list.remove("a")
messy_list.remove(False)
messy_list.remove([1, 2, 3])
Lisa Sparks
3,187 Pointsthe_ list.remove{1,2,3) don't use the #1
Lisa Sparks
3,187 PointsLisa Sparks
3,187 Pointsdon't use #1 in list