Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial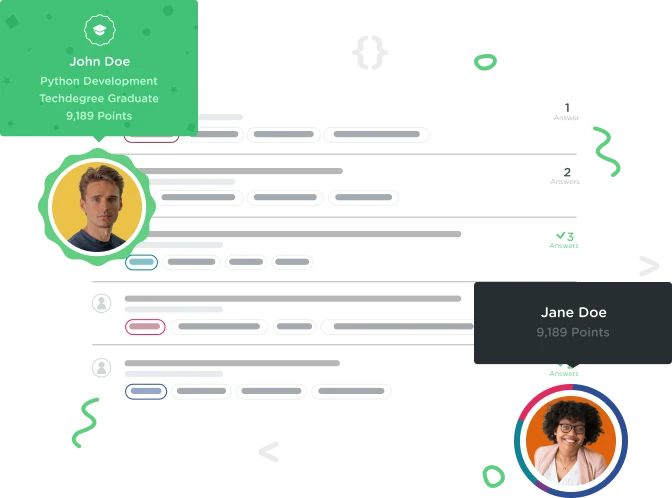

Samuel Andrei Daniel Goje
1,351 PointsHow do I remove list members from a list? (It doesn't seem to work as I thought).
I can't figure out what I did wrong/what I should do to fix it.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
a = the_list.pop(3)
the_list.insert(0 , a)
the_list.remove(False)
the_list.remove("a")
del the_list(3)
del the_list(4)
del the_list(5)
3 Answers
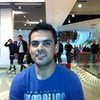
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Samuel
I did it like this
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0,the_list.pop(3))
the_list.remove("a")
del the_list[3:len(the_list)] # I used list slice here to get rid of index 3 and 4. You could also do it like del the_list[3:] that would work to as it would go till the end of the array
even though its a list member it still has an index so just use del listname[the index]

Charlie L.
10,120 PointsThe reason your original code doesn't work is that every time you use "del" to delete a list item, the list is recreated which means the indexes are also changed.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
del the_list(3) # returns a list of: ["a", 2, 3, False, [1, 2, 3]]
del the_list(4) # returns a list of: ["a", 2, 3, False], note that the removed item is not the fourth item in the original but from the fourth index of the new list.
del the_list(5) # now you can see that there is no index 5 list item which results in the index error.
Hope this helps!

Samuel Andrei Daniel Goje
1,351 PointsThanks!
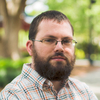
Kenneth Love
Treehouse Guest TeacherAlso you don't delete indexes with ()
, you delete them with []
.
Samuel Andrei Daniel Goje
1,351 PointsSamuel Andrei Daniel Goje
1,351 PointsCan you please explain how del the_list[3:len(the_list)] this works? (as far as i'm know del only needs one value and that is the index value and I also don't knonw what 3:len(the_list) would do)
Thanks in advance!
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsAndreas cormack
Python Web Development Techdegree Graduate 33,011 Pointsnormally a list will take an index as its argument but you an also slice chunks out from an array hence the concept of slicing comes in. Once you go through slicing in the course this code will be more understandable. when 1 is moved to the front and a is removed we end up with a list [1,2,3,False,[1,2,3]] where False is at index 3 and [1,2,3] is at index 4 hence i sliced out or deleted False and [1,2,3] by passing a range del the_list[3,len(the_list)]. The function len() returns the length of the list which is 4. I could have also just written it like so del the_list[3:4] that would remove values at index 3 and index 4.
Hope that helps