Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial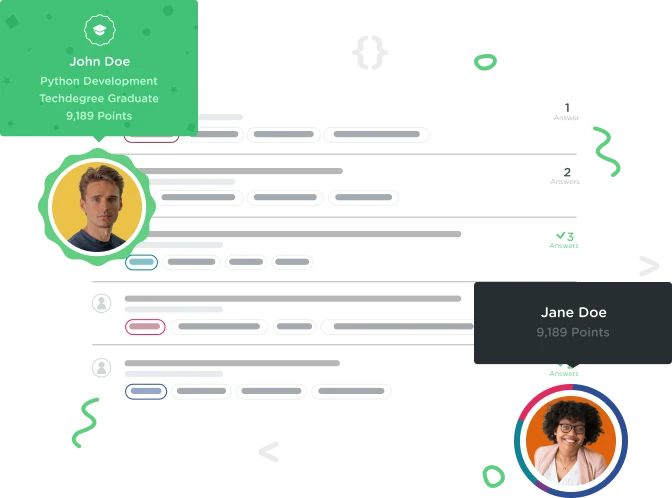
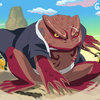
Neerav Aiyappa
2,024 PointsHow do I retrieve a value of an array and compute it to a constant
this problem appeared in one of my code challenge
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers.count)
counter++
}
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey there!
Values of an array at a certain index can be retrieved via subscripting. See Apple Swift docs: Accessing and Modifying an Array. I have added the approach with comments below.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Loop as long as counter is less than the number of Ints in the array.
// Why less than? Arrays are zero based, in our case valid indices are
// 0, 1, 2, 3, 4, 5, 6. Count will give you seven, as there are seven Ints in our array
while counter < numbers.count {
// Add the value of the Int at the index `counter` to `sum`
// You can access values of an array at a specific index via subscripting,
// array[index]. You should always make sure that the index exists. If your
// index is out of range, this will result in a crash
sum += numbers[counter]
// Increment counter by one, otherwise we will end up
// with an infinite loop. Please note that `++` and `--`
// are deprecated in Swift 3. See the example below for the
// Swift3 version.
counter++
}
// ...
while counter < numbers.count {
sum += numbers[counter]
counter += 1
}
Hope that helps :)