Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial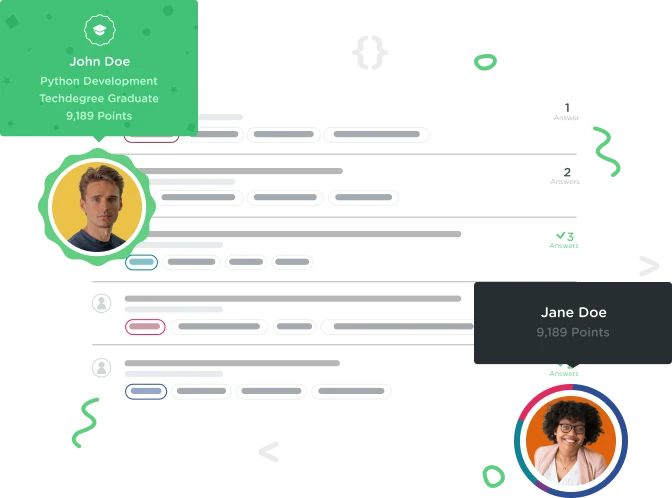
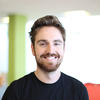
Kristian Woods
23,414 PointsHow do I retrieve data from a database using a search bar?
I have set up my local host database. I'm trying to pull data from the database, using PHP. However, I'm running into never-ending errors.
<?php
// Create a database connection
$dbhost = "localhost";
$dbuser = "root";
$dbpass = "root";
$dbname = "music_online";
$connection = mysqli_connect($dbhost, $dbuser, $dbpass, $dbname);
// 1. Test if connection occurred
if(mysqli_connect_errno()) {
die("Database connection failed: " . mysqli_connect_error() . " (" . mysqli_connect_errno() . ")");
}
?>
<?php
// 2. perform a database query
$cleanSearch = mysqli_real_escape_string($_POST['search']);
$query = mysqli_query("SELECT * FROM music_info WHERE artist LIKE '%$cleanSearch%'");
// test if there was a query error
if(!$query) {
die("Database query failed");
}
?>
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="main.css">
</head>
<body>
<h1>Music Info</h1>
<form method="POST" action="index.php" id="searchForm">
<input type="text" id="searchBox" name="search" placeholder="search...">
<input type="submit" name="submit" value="search">
</form>
<?php
// 3. use returned data (if any)
while($row = mysqli_fetch_row($query)) {
// output data from each row
var_dump($row);
echo "<hr/>";
}
?>
<?php
// 4. release returned data
mysqli_free_result($query);
?>
</body>
</html>
<?php
// 5. Close database connection
mysqli_close($connection);
?>
I continue to get the error "Database query failed"
Thanks
1 Answer
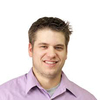
Kevin Korte
28,149 PointsWell, we know that that query variable is coming back false - so one of two things are happening to check for. Either our query really is coming back false, or we're not connected to the database. Do you have phpmysql or mysql workbench running so you can run queries?
I would first run you query directly on the database, and see what you get back. Once you know that a search term should return you a result, start backing out each line of code until you find the culprit. Make sure your cleansearch variable is being populated like you expect, make sure your connection is being created like you expect. Simple var_dumps should provide enough info for you to know.
Let us know how that goes.
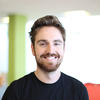
Kristian Woods
23,414 PointsHey, Kevin, thanks for getting back to me. I ran the query in phpmyadmin, and replaced the search variable with a value I know should generate returned data. Yet, I still doesn't work when I use it through the search box.
The error that I receive, pops up as the page loads... before I've even had the chance to type anything into the search box.
I removed this line of code, and the error stopped displaying. But I still can't return results from the database
// test if there was a query error
if(!$query) {
echo "No results found";
}
Diar Selimi
1,341 PointsDiar Selimi
1,341 PointsAdd an if condition in your query, i think the query is trying to show something maybe when you are entering the page for the first time !!