Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial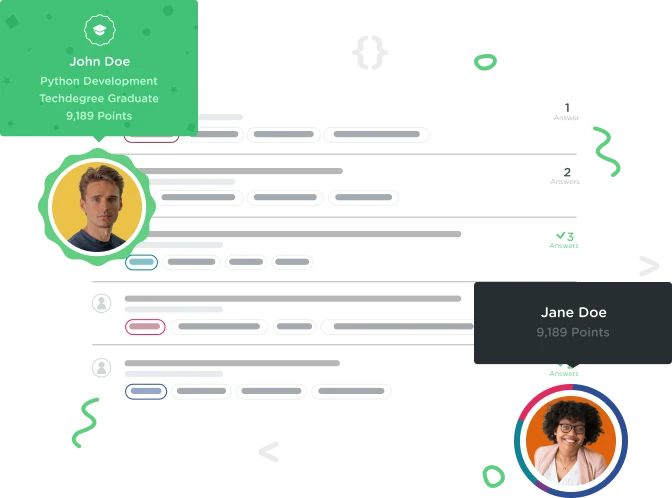
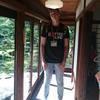
Charles Roennfeldt
1,163 PointsHow do I retrieve each value from an array and add it to the value of my variable 'sum'? Using counter as an index value
Can anyone please help me with how I am to approach this? I am stuck trying to work out how to retrieve each individual value and add them together using a while loop.
2 Answers
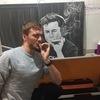
Tobias Mahnert
89,414 PointsHi Charles,
the Code below solves the Challenge, if you want to know why, lease let me know.
Cheers Toby
also, please rate my answer as best answer if it helped you .
while counter < numbers.count {
sum += numbers[counter]
counter++
}

Martin Wildfeuer
Courses Plus Student 11,071 PointsThe idea is to use a while loop and run the loop as long as there are numbers in the array. With every iteration, we would receive the value from the numbers array, add its value to sum and then increment the counter.
This approach is based on two ideas:
- You get an element of an array by providing its index:
numbers[index]
- The while loop runs only if there are still numbers in the array. We can get the number of elements an array contains via
count
So, a while loop runs as long as a condition is true. In our case, the condition would be
// If counter is less than the number of elements in the numbers array, this is going to be true
counter < numbers.count
Remember, array indices are zero based, that is the index of the first element is 0.
count
will return the number of elements in an array, so if there are 4 elements,
the index of the last element is 3.
Therefore, if you want to run a while loop as long as there are numbers in the array
// If counter is less than the number of elements in the numbers array, this is going to be true
while counter < numbers.count {
counter++
}
See how we increment the value of counter every time the loop runs? This way we make sure it runs just as often as there are elements in numbers and it won't run infinitely.
The only thing left to do now is getting the value for each element. As mentioned before, we can get an element of an array by it's index. As we are incrementing counter
one by one, we can use counter to get each element:
numbers[counter]
So the final code would look like this:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
while counter < numbers.count {
sum += numbers[counter]
counter++
}
Hope that helped :)
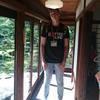
Charles Roennfeldt
1,163 PointsAwesome thanks! Clears up the confusion

Dean Kreseski
2,418 Pointsthis helped tremendously man thanks
Charles Roennfeldt
1,163 PointsCharles Roennfeldt
1,163 PointsSweet! Thanks dude