Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial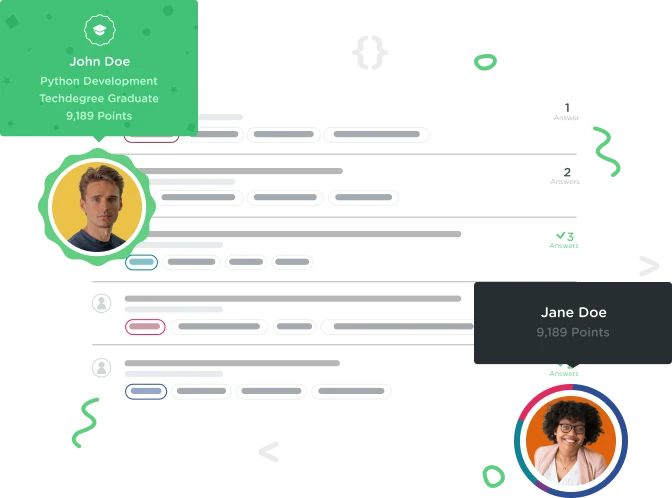

angel angel
6,620 Pointshow do I retrieve the information from one page to the next page with php and SQL query to update?
So This is the code for my application but the thing that is not working is when I submit the information the information is not showing in the other page.
php
index.php
<?php
require_once('database.php');
// Get category ID
if (!isset($category_id)) {
$category_id = filter_input(INPUT_GET, 'category_id',
FILTER_VALIDATE_INT);
if ($category_id == NULL || $category_id == FALSE) {
$category_id = 1;
}
}
// Get name for selected category
$queryCategory = 'SELECT * FROM category
WHERE categoryID = :category_id';
$statement1 = $db->prepare($queryCategory);
$statement1->bindValue(':category_id', $category_id);
$statement1->execute();
$category = $statement1->fetch();
$category_name = $category['categoryName'];
$statement1->closeCursor();
// Get all categories
$query = 'SELECT * FROM categories
ORDER BY categoryID';
$statement = $db->prepare($query);
$statement->execute();
$categories = $statement->fetchAll();
$statement->closeCursor();
// Get products for selected category
$queryProducts = 'SELECT * FROM products
WHERE categoryID = :category_id
ORDER BY productID';
$statement3 = $db->prepare($queryProducts);
$statement3->bindValue(':category_id', $category_id);
$statement3->execute();
$products = $statement3->fetchAll();
$statement3->closeCursor();
?>
<!DOCTYPE html>
<html>
<!-- the head section -->
<head>
<title>My Guitar Shop</title>
<link rel="stylesheet" type="text/css" href="main.css" />
</head>
<!-- the body section -->
<body>
<header><h1>Product Manager</h1></header>
<main>
<h1>Product List</h1>
<aside>
<!-- display a list of categories -->
<h2>Categories</h2>
<nav>
<ul>
<?php foreach ($categories as $category) : ?>
<li><a href=".?category_id=<?php echo $category['categoryID']; ?>">
<?php echo $category['categoryName']; ?>
</a>
</li>
<?php endforeach; ?>
</ul>
</nav>
</aside>
<section>
<!-- display a table of products -->
<h2><?php echo $category_name; ?></h2>
<table>
<tr>
<th>Code</th>
<th>Name</th>
<th class="right">Price</th>
<th> </th>
<th> </th>
<th> </th>
</tr>
<?php foreach ($products as $product) : ?>
<tr>
<td><?php echo $product['productCode']; ?></td>
<td><?php echo $product['productName']; ?></td>
<td class="right"><?php echo $product['listPrice']; ?></td>
<td>
</td>
<td><form action="delete_product.php" method="post">
<input type="hidden" name="product_id"
value="<?php echo $product['productID']; ?>">
<input type="hidden" name="category_id"
value="<?php echo $product['categoryID']; ?>">
<input type="submit" value="Delete">
</form></td>
<td><a href="update_form.php?product_id=<?php echo $product['productID'];?>">Edit</a></td>
</tr>
<?php endforeach; ?>
</table>
<p><a href="add_product_form.php">Add Product</a></p>
<p><a href="category_list.php">List Categories</a></p>
</section>
</main>
<footer>
<p>© <?php echo date("Y"); ?> My Guitar Shop, Inc.</p>
</footer>
</body>
</html>
update_form.php
<?php
require('database.php');
$product_id = $_GET['product_id'];
$query = 'SELECT *
FROM products
where productID = :product_id';
$statement = $db->prepare($query);
$statement ->bindValue(':product_id', $product_id);
$statement->execute();
$product = $statement->fetch();
$statement->closeCursor();
// Get all categories
$query = 'SELECT * FROM categories
ORDER BY categoryID';
$statement = $db->prepare($query);
$statement->execute();
$categories = $statement->fetchAll();
$statement->closeCursor();
?>
<!DOCTYPE html>
<html>
<!-- the head section -->
<head>
<title>My Guitar Shop</title>
<link rel="stylesheet" type="text/css" href="main.css">
</head>
<!-- the body section -->
<body>
<header><h1>Product Manager</h1></header>
<main>Edit Product</h1>
<form action="update_product.php" method="post"
id="update_form">
<label>Category:</label>
<select name="category_id">
<?php foreach ($categories as $category) : ?>
<option value="<?php echo $category['categoryID']; ?>">
<?php echo $category['categoryName']; ?>
</option>
<?php endforeach; ?>
</select><br>
<label>Code:</label>
<input type="text" name="code" value="<?php echo $product['productCode']; ?>" ><br>
<label>Name:</label>
<input type="text" name="name" value="<?php echo $product['productName']; ?>"><br>
<label>List Price:</label>
<input type="text" name="price" value="<?php echo $product['listPrice']; ?>"><br>
<label> </label>
<input type="submit" value="Edit">
</form>
<p><a href="index.php">View Product List</a></p>
</main>
<footer>
<p>© <?php echo date("Y"); ?> My Guitar Shop, Inc.</p>
</footer>
</body>
</html>
update_product.php
<?php
// Get the product data
$category_id = filter_input(INPUT_POST, 'category_id', FILTER_VALIDATE_INT);
$code = filter_input(INPUT_POST, 'code');
$name = filter_input(INPUT_POST, 'name');
$price = filter_input(INPUT_POST, 'price', FILTER_VALIDATE_FLOAT);
// Validate inputs
if ($category_id == null || $category_id == false ||
$code == null || $name == null || $price == null || $price == false) {
$error = "Invalid product data. Check all fields and try again.";
include('error.php');
} else {
require_once('database.php');
// Add the product to the database
$query = "UPDATE products
SET categoryID = :category_id,
productCode = :code,
productName = :name,
listPrice= :price
WHERE categoryID = :category_id , productCode = :code ,productName = :name,
listPrice = :price ";
$statement = $db->prepare($query);
$statement->bindValue(':category_id', $category_id);
$statement->bindValue(':code', $code);
$statement->bindValue(':name', $name);
$statement->bindValue(':price', $price);
$statement->execute();
$statement->closeCursor();
// Display the Product List page
include('index.php');
}
?>