Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial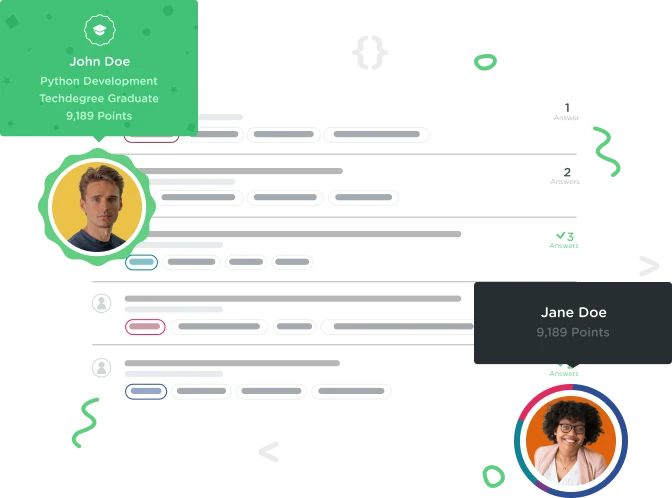
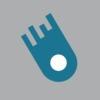
Gabriel E
8,916 PointsHow do I return 0 for equality?
Hi there,
I'm working on an equality challenge, and I have completed the first task, but the 2nd and 3rd is giving me problems. Here is my code, can anyone help? Thanks!
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(Object obj) {
return 1;
if (obj == 0) {
return obj.compareTo(BlogPost.obj);
}
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
3 Answers
Christopher Augg
21,223 PointsGabriel,
You will want to use the this keyword as it is a reference variable that refers to the current object. It will allow you to test for equality between this object and the one being passed into the compareTo(Object obj) method after you complete the casting of a BlogPost. You should use the equals() method to do so.
Some hints for Task 2/3
@Override
public int compareTo(Object obj) {
// declare a BlogPost named post and assign the cast of obj to it.
// test if **this** equals(post) and return 0 if it does
// said to keep return 1 for now.
return 1;
}
Hints for 3/3: Once you have the code completed for 2/3, you want to only change the return 1 statement here to return a comparison of this creation date with the creation date of post. Use the compareTo() method to do so. Remember, the compareTo() method you are writing is your Overridden version of it; however, calling compareTo() inside your implementation would be calling the Object's compareTo() method. In this case, that would be a Date object that implements Comparable and has it's own compareTo() method.
@Override
public int compareTo(Object obj) {
// declare a BlogPost named post and assign the cast of obj to it.
// test if **this** equals(post) and
// return 0 if it does
//return **this** date using compareTo(post's date);
}
Regards,
Chris

Mahmoud Yousif
5,053 PointsOK so lets tackle the second one first. You pass java object that is actually a BlogPost in the method so you need to make it into a BlogPost to be able to compare it. And you compare it to the original object by using the equals method. I'm going to show you an example of how Craig uses this with Treets, which is the same concept
public int compareTo(Object obj){
//So we need to make the object into a Treet so we can compare it:
Treet treet = (Treet) obj; //This line of code stores the obj information into a Treet
//We can do that since Treet is a child of Object
//next we need to check if it equals the original object which we can do with the equals() method
//the method returns a boolean(true or false) so we can pass it in an if statement
if(equals(treet)){
return 0; //so this means if the equals method returns true then it will return 0
}
return 1;//we return a 1 as a default just in case the condition didn't work out
}
With the third one, we need to compare the creationDate of the treet so instead of the default 1 being returned, we can return an actual comparison based on the earlier post.
public int compareTo(Object obj){
Treet treet = (Treet) obj;
if(equals(treet)){
return 0;
}
//So we need to call the compareTo() method to compare the dates
//but this returns an int so we need to store it to see the result
int compare = mCreationDate.compareTo(treet.getCreationDate);
//so whatever gets returned in the compareTo method will be the comparison we need
//so lets return it so we know which one came first based on the response
return compare;
}
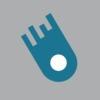
Gabriel E
8,916 PointsThanks Mahmoud,
Last night I just figured it out. I think my way was a bit more involved and unnecessarily long, but it worked and I understand it now. Thanks!

Mahmoud Yousif
5,053 PointsI went back and went through the challenges to refresh myself on it. I'll post some more detailed instructions. If you need a code example let me know and i'll post one too.
Second Challenge: -First, you need to cast the object you passed into your compareTo method into a BlogPost. To do that just create a new BlogPost and declared as the casted object. -Second, you need to create an if statement that checks if the objects equal each other, using the new BlogPost you created. And return 0 if that is the case. -Third, the return 1 statement needs to be after the if statement. To return 1 if the condition isn't true.
Third Challenge: -First, you need to get rid of your default return statement that returns 1. -Second, create an int to store the comparison of your mCreationDate of the current blog post and the post you created by casting the object. -Third, return the int you created to check equality.
Feel free to ask me any other questions. Hope this helps :).
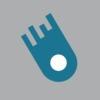
Gabriel E
8,916 PointsHi Mahmoud,
Thanks for the help! I was wondering if you could post a code example, just so I could get the feel of what I should be doing. Thank you!
Gabriel