Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial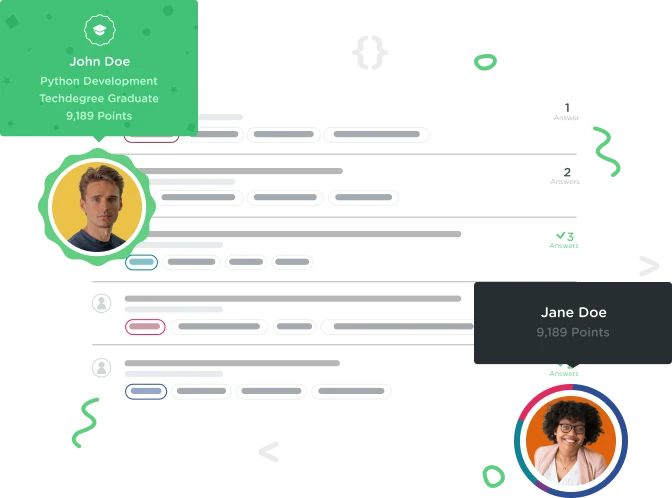

James Casavant
21,522 PointsHow do I return a boolean when an array is empty?
This is the question I'm currently working with:
In the TodoList class, fill in the empty? method to return a boolean value. The empty? method should return true if there are no elements in the @todo_items array. The method should return false if there is more than one element in the @todo_items array.
Here is the code as it was:
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def empty?
if @todo_items = nil
return true
else
return false
end
end
and one of my solutions:
def empty?
if @todo_items = nil
return true
else
return false
end
I've tried "[]" "[""]" "0"
I've also tried: @todo_items.nil? @todo_items.any?
9 Answers
William Li
Courses Plus Student 26,868 PointsFirst, you can NOT check if an Array is empty by checking it against nil
[] == nil # => false
This doesn't work, empty Array doesn't equal to nil
Now for you problem, you can make use of the Array build-in method empty?
to do the job http://ruby-doc.org//core-2.2.0/Array.html#method-i-empty-3F
def empty?
todo_items.empty?
end
alternatively, you can tell if Array is empty by checking its length
def empty?
todo_items.length == 0
end
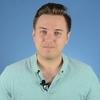
Hunter Garrett
6,339 PointsI wrote
def empty?
if todo_items.length == 0
return true
elsif todo_items.length > 1
return false
end
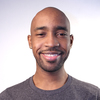
David Tonge
Courses Plus Student 45,640 PointsI think your problem is that you're assigning instead of comparing with your conditional statements. "=" vs. "==" look at your if statements

James Casavant
21,522 PointsThank you both for your answers. I actually was missing an "end". :|
William, thank you for the additional information about nil.

Darrell Padua
7,895 PointsYou don't need to use the @ for the instance variable since todo_items is already defined in the attr_reader method.

Kasia Ekiert
Courses Plus Student 13,248 Pointsdef empty? if @todo_items != [] empty = false else empty ||= true end end

Carlos Carrillo
15,596 PointsI did it like this:
if @todo_items == [] return false else return true end
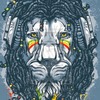
eddyak
5,830 Pointsdef empty?
answer = false
if todo_items.length == 0
answer = true
end
return answer
end
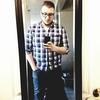
Aaron Cleveland
1,971 Pointsclass TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def empty?
@todo_items == [] ? true : false
end
end
William McManus
14,819 PointsWilliam McManus
14,819 PointsThat first bit was brilliant! Guess there was a hint in the Array name huh:)