Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial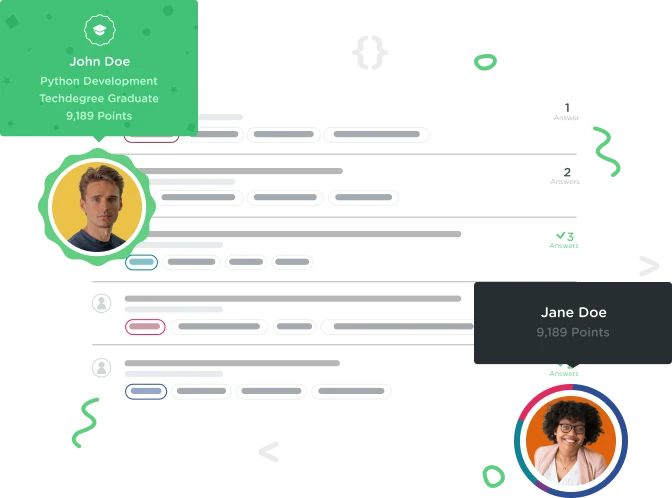

Roman Casal
10,096 PointsHow do I select only the Elements iniside of an specifict html tag?
For explample, if i want only to select the list items elements inside of an nav tag, How can I do it?
let navigationLinks = document.getElementsByClassName("selected");
let galleryLinks;
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html" class="selected">About</a></li>
<li><a href="contact.html" class="selected">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>

Roman Casal
10,096 PointsThanks Gustavo !!
1 Answer
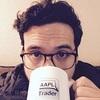
Nicholas Gaerlan
9,501 PointsI remember this one. Not your fault here, the videos DO NOT EXPLAIN a feature of the querySelectorAll(). Turns out you can do this...
let navigationLinks = document.querySelectorAll('nav a');
in fact, there's pretty much no reason to use anything but the querySelector or querySelectorAll at this stage. Then again, I've sort of learned that you are learning this stuff to keep in the back of your mind because later you learn about different libraries that simplify all of this and more, so out in the wild, you'll rarely have to resort to these methods. You still have to learn them... but I think this is all just groundwork before you learn JQuery... then for other things you're gonna learn Node and React or a framework like Angular. I'm not there yet, but... that's my feeling

Roman Casal
10,096 PointsThanks a lot! that was exactly what happened to me, and yes the video does not explain it -.-...
Gustavo Winter
Courses Plus Student 27,382 PointsGustavo Winter
Courses Plus Student 27,382 PointsHello Ricardo, to select elements inside a html tag, you will need to select the parent node first and then the children.
Like this:
As we know, the DOM is like a tree, you always can select the children if you target the correct parent.
By the way, in this case i use querySelector but you can also use getElementById.
I suggest you to read these articles for better understanding:
Children
JavaScript HTML DOM Elements
Obs: Use console.log(); and walk throught the DOM, using the tools provide at this course JavaScript and the DOM