Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial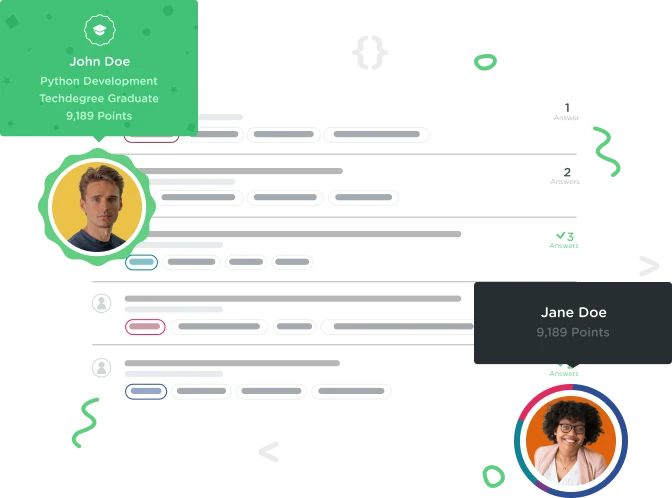
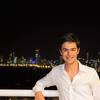
SERGIO RODRIGUEZ
17,532 PointsHow do I set default values to properties that where not specified during the instance creation?
In php, How do I set default values to properties that where not specified during the instance creation?
I have this code:
class Product
{
public $name = 'default name';
public $price = 0;
public $desc = 'default description';
function __construct($name, $price, $desc) {
$this->name=$name;
$this->price=$price;
$this->desc=$desc;
}
public function getInfo(){
return $this->name;
}
}
$p = new Product();
var_dump($p);
The problem is $p has NULL on all 3 parameters, instead of having 'default name', 0 and 'default description'.
Thanks!
3 Answers
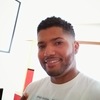
Emmanuel Salom
Courses Plus Student 8,320 PointsWith function __construct you are instructing that the instance of the class must have $name, $price, and $description. So every time you create a new object you must provide the 3 parameters as per the instructions in the __construct method. You can create the new instance of the class and set the values without the constructor. ex. $p->name = 'new name'. If you don't set the new value then will be $p->name = 'default name'.
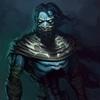
Adrian Buenache
Python Web Development Techdegree Graduate 18,696 PointsYou can also set default values inside the construct function using an if statement, this way if you forget to set one of the values, or create a new object with no arguments you get a default product:
<?php
class Product {
public $name;
public $price;
public $desc;
function __construct($name, $price, $desc) {
if(!$name || !$price || !$desc) {
$this->name = 'default name';
$this->price = 0;
$this->desc = 'default description';
} else {
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
}
public function getInfo() {
return "Product name: $this->name";
}
}
$p = new Product();
echo $p->getInfo();
?>
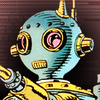
Robert Smith
8,485 PointsIf you wanted to set values after the product creation you can also use a setter method instead of passing default values when the object is created:
<?php
class Product
{
public $name = 'default name';
public $price = 0;
public $desc;
function __construct($name, $price, $desc) {
$this->name=$name;
$this->price=$price;
$this->desc=$desc;
}
public function getInfo(){
return $this->name;
}
public function setInfo(desc){
$this->desc=desc;
}
}
//Create the product object
$p = new Product();
//call the setter method and pass it a description
$p->setInfo($desc);
?>