Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial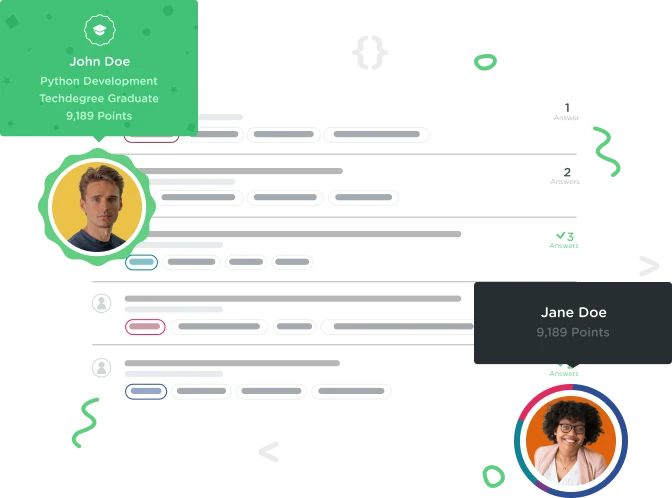

Niem Nguyen
2,502 PointsHow do I show my result (from scripts) on a <li> on my webpage?
This is my HTML
<body>
<div class="container">
<h1>Ping Pong</h1>
<form id="pongTable">
<div class="form-group">
<label for="pingPong">Enter number to start game: </label>
<input id="userInput" type="number">
</div>
<button type="submit" class="btn btn-success">Play!</button>
</form>
<div>
<ul class="result"></ul>
</div>
</div>
</body>
Here is the logic
//BUSINESS LOGIC
var pingPong = function(userInput) {
for (var i = 1; i < userInput; i++) {
if ((i % 3 === 0) && (i % 5 ===0)){
document.write("ping pong");
} else if (i % 5 === 0) {
document.write("ping");
} else if (i % 3 === 0) {
document.write("pong");
} else {
document.write(i);
}
}
}
//UI LOGIC
$(function() {
$("#pongTable").submit(function(event) {
event.preventDefault();
var userInput = parseInt($("#userInput").val());
var result = pingPong(userInput);
$(".result").text(result);
});
});
This is what I get when I enter a number like 15: 12pong4pingpong78pongping11pong1314
I would like it to look like a list of list items instead of this one giant run-on.
2 Answers
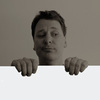
Sean T. Unwin
28,690 PointsThis is the way I completed the task. I have commented the changes that have been made, but if you have questions feel free to ask. :)
//BUSINESS LOGIC
// Create a list item as a string and return it
// Takes 1 argument which is the content to display in the list item
function createLi(content) {
// opening list item tag
var openLi = '<li>';
// closing list item tag with line ending
var closeLi = '</li>\n';
// return the content enclosed in a list item
return openLi + content + closeLi;
}
var pingPong = function(userInput) {
// String to hold all the list items
var str = '';
for (var i = 1; i < userInput; i++) {
if ((i % 3 === 0) && (i % 5 ===0)){
// Create list item with message
// Add list item to string
str += createLi("ping pong");
} else if (i % 5 === 0) {
// Create list item with message
// Add list item to string
str += createLi("ping");
} else if (i % 3 === 0) {
// Create list item with message
// Add list item to string
str += createLi("pong");
} else {
// Create list item with message
// Add list item to string
str += createLi(i);
}
}
// Return the string
return str;
}
//UI LOGIC
$(function() {
$("#pongTable").submit(function(event) {
event.preventDefault();
var userInput = parseInt($("#userInput").val());
var result = pingPong(userInput);
// Changed .text() to .html() for rendering the li tags
$(".result").html(result);
});
});
All that is left to do is to style the list. :-D

Niem Nguyen
2,502 PointsThank you! It looks very comprehensive. Much appreciated =)

Arjuna Huffman
2,400 PointsIf you want to append list items to an unordered list, you can do that like this:
//HTML markup
<ul id="some-list"></ul>
//JavaScript
var names = ['Bill', 'Selma', 'Henry the fifth']; var someList = document.getElementById('some-list');
function addToList(list, arr) {
/* We append the list items to a document fragment first so as to minimize the amount of work we do on the DOM.
A document fragment is like a virtual dom node that you can append items too and then, to insert the new markup into the DOM, you simply append the document fragment to it. */
var fragment = document.createDocumentFragment();
for (var i = 0; i < arr.length; i++) {
//Create the list item
var listItem = document.createElement('li');
//Set the text content of the list item
listItem.textContent = arr[i];
//Append the list item to the document fragment
fragment.appendChild(listItem);
}
//When the loop is over, append the document fragment to the dom.
list.appendChild(fragment);
}
addToList(someList, names);

Niem Nguyen
2,502 PointsThanks for the tips. I will test it out!
Sean T. Unwin
28,690 PointsSean T. Unwin
28,690 PointsI edited your post for code formatting.