Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial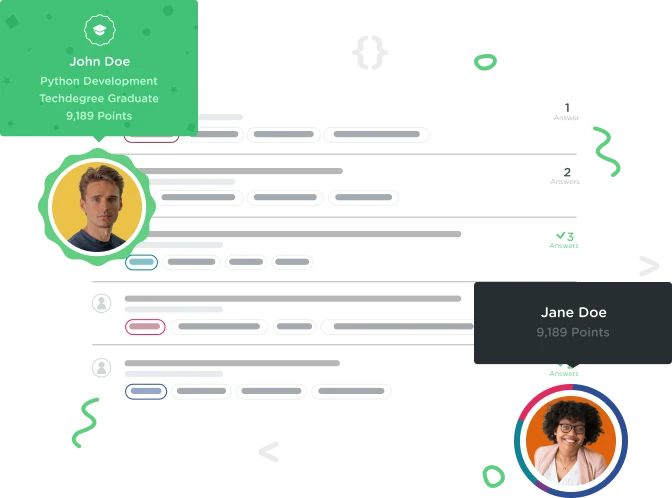

Alex Fernandez
1,281 PointsHow do I solve this challenge?
Where do I go. The way the challenge is described confuses me. Thanks ahead of time.
func isDivisible(#dividend: Int, #divisor: Int) -> Bool {
if dividend % divisor == 0 {
return true
} else {
return false
}
}
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
if dividend % divisor != 0{
return false
}
}
2 Answers
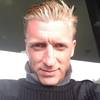
Rasmus Rønholt
Courses Plus Student 17,358 PointsHey Alex... You are on the right path, but as your isNotDivisible function is implemented now, it will not compile, since you are only returning a value (false) when it is NOT divisible... Now to fix it so it will compile, you should return a value in all circumstances - you have to do this every time you implement a function with a return value. Do you understand?
Now for the task itself, it seems the concept confuses you a bit. When running the function isNotDivisible you would expect to get a value of true if the numbers are in fact NOT divisible - but you return false. But try to always think about reusing your code. In this case you already have a function that returns a boolean value of whether or not the numbers are divisible, so your new isNotDivisible should always return the exact opposite. When working with booleans this is quite simple, and you can do the following:
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
return !isDivisible(dividend: dividend, divisor: divisor)
}
Nb. Note the "!" in front of the function call, which is what inverts the boolean that we get back from isDivisible :)
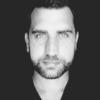
Jhoan Arango
14,575 PointsHello Alex:
You were on your way there, all you were missing was the right returns. Remember that when using conditional statements the IF statement in its simplest form has a single if condition, and it will execute a set of statements only if the condition is true.
// These two examples are IF statements it it’s simplest form
if 2 > 1 {
// It will execute because is true that 2 is higher than 1
}
if 1 > 2 {
// This will not execute because is NOT true that 1 is higher than 2
}
“The if statement can provide an alternative set of statements, known as an else clause, for when the if condition is false. These statements are indicated by the else keyword:”
I recommend reading. “The Swift Programming Language (Swift 2 Prerelease).” iBooks. https://itun.es/us/k5SW7.l
if 1 > 2 {
// It will skip this since its not true
} else {
// it will execute because it is false
}
Here is the answer to your challenge.
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
if dividend % divisor != 0 {
return true
} else {
return false
}
}
From what I saw on your code, it seems as if you didn’t understand the conditional statement part, that’s why I gave you this answer, if your confusion was the return concept, then please let me know and I can try to explain that better.
Hope this answer helps you.