Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial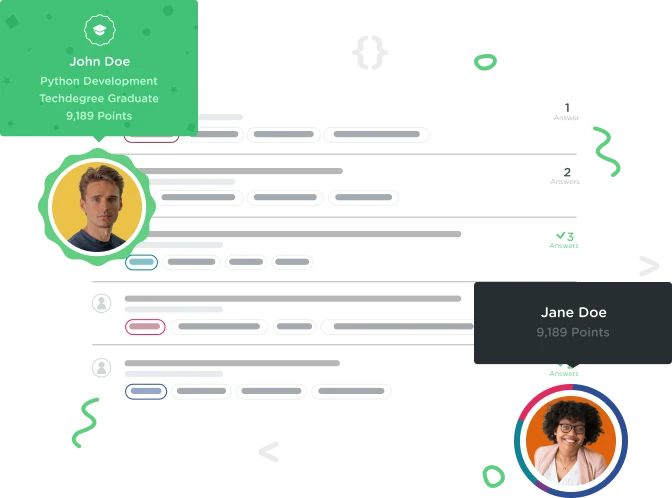

Melissa Benton
Front End Web Development Techdegree Graduate 17,805 PointsHow do I specify, in the condition statement, which li element I want to be emboldened?
I have been able to write a conditional statement that will embolden all the li elements when a 0, 1 or 2 is typed in the input field. However, I cannot figure out how to specify that the li element corresponding to the index value be emboldened only.
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index === 0 ) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
2 Answers

eotfofiw
Python Development Techdegree Student 824 PointsLet's work our way in from the event listener and see if we can get there.
The listener is what's responding to us clicking the embolden button on the form. We're not using any of the data stored on the incoming event object (e). We can move on.
We're getting the value of the user provided input field next.
// A reminder of what indexText is
// const indexText = document.getElementById('boldIndex');
const index = parseInt(indexText.value, 10);
indexText is live. Whenever the event handler runs on click, we're getting the value of what's in that input field. So when the user updates it, and the event handler fires, we will get the current value of it.
Let's assume that the user has entered the value 1
in that text input. It's really worth remembering that this index
(which could probably be named something better) is what represents what the user has typed in to the input and that translates to the index in the list they want to embolden.
That last part if key to figuring out what to add to the condition.
Next we loop over the list of laws (the list of li
elements) by their index. We're grabbing the law element from that list by index, but it's only important to set styling later. The condition doesn't care about it. The condition cares about 2 things:
- The index the user has said they want to embolden
- Which index are we at in the list of
li
elements (laws)
If the user has said 1
should be emboldened, and we're on our first element in the list (which is the zero index) then we're at 0, the user said they want 1 emboldened. We should not make it bold.
If the user has said 1
should be emboldened, and we're on our second element in the list (which is the 1 index) then we're at 1, the user said they want 1 emboldened. We should make it bold.
If the user has said 1
should be emboldened, and we're on our third element in the list (which is the 2 index) then we're at 2, the user said they want 1 emboldened. We should not make it bold.
Hopefully that gives you an idea of what values you should be comparing in the if statement - without just giving you the answer outright.
Good luck!
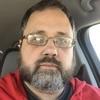
Mark Sebeck
Treehouse Moderator 38,304 PointsHi Melissa. So you have half of it. Index is the value they type in. As the for statement runs to built the li elements it will equal 0,1 then 2. So you want to bold it when index equals i. Hope this helps. Good luck!