Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial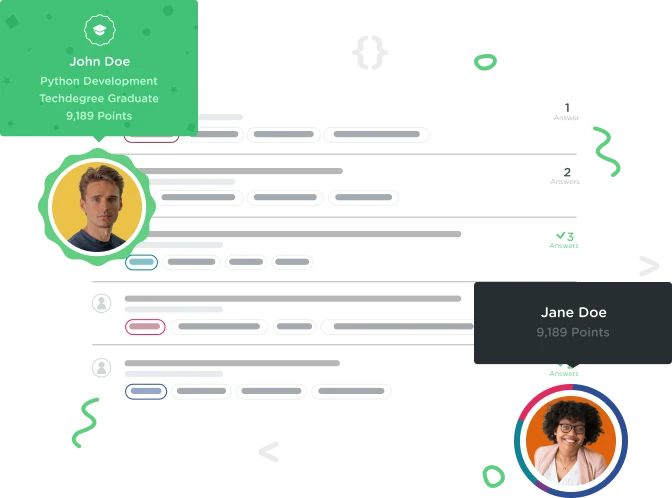

chazel
Courses Plus Student 6,961 PointsHow do I style three divs as two columns with either flex or css-grid?
Goal
I want two divs (title and description) in column 1 with one div (button) in column 2 (see Layout Goal for a visual example using table markup). The button should be vertically aligned to the center of the first column if that column grows taller than column 2. I only want to use CSS to create the layout--either css-grid or flexbox, and I do not want to inherit any of the grid styling from the outer grid.
What I Have Tried
I have tried a variety of css-grid and flex layouts, and none of them worked. I could not figure out flexbox because I could never find a layout that was similar to my end goal, but it felt like it may be what I need. The closest I was able to get was using css-grid, but the second column would either grow (grid-row: 1/3) too much, making the whole column the button, or the button would not center vertically.
Samples
- Target Code: https://codepen.io/u1138/pen/JjzpzMb
- Layout Goal (using a table): https://codepen.io/u1138/pen/MWxQxEN
.outer-grid {
display: grid;
grid-template-columns: repeat(3, 1fr);
column-gap: 24px;
row-gap: 24px;
}
.container {
padding: 24px;
border: 1px solid black;
}
.title {
font-weight: bold;
font-size: 1.2em;
margin-bottom: 12px;
}
.button {
display: inline-block;
padding: 12px;
background-color: lightgray;
border: 1px solid darkgray;
}
/* flex/grid properties */
.container {
}
.title,
.description {
}
.button {
}
<div class="outer-grid">
<div class="container">
<div class="title">Title of Element</div>
<div class="description">This is the description of the element.</div>
<a class="button">Button</a>
</div>
<div class="container">
<div class="title">Title of Element</div>
<div class="description">This is the description of the element.</div>
<a class="button">Button</a>
</div>
</div>
2 Answers
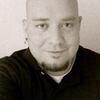
Brian Jensen
Treehouse StaffHiya chazel!
With the way your HTML is currently set up, this is what I would recommend with CSS Grid:
.container {
display: grid;
grid-template-columns: 1fr max-content;
grid-template-rows: fit-content(100%);
grid-template-areas:
"title button"
"description button";
column-gap: 24px;
}
.title{
grid-area: title;
}
.description {
grid-area: description;
}
.button {
grid-area: button;
align-self: center;
}
HOWEVER, I would highly recommend that you modify the HTML for the container div to be this structure. So that it only has two direct children instead of three:
<div class="container">
<div class="text">
<div class="title">Title of Element</div>
<div class="description">This is the description of the element.</div>
</div>
<a class="button">Button</a>
</div>
Then you will be able to use Flexbox with more control and simplified CSS syntax to achieve it with just rules on the container div alone:
/* flex/grid properties */
.container {
display: flex;
gap: 24px;
justify-content: space-between;
align-items: center;
}

chazel
Courses Plus Student 6,961 PointsBrian Jensen Thank you very much! That works great, and I agree that modifying the markup would be the best course. Unfortunately, I cannot. :(
If I use the following on .container
, then I can get the interior contents centered top-to-bottom, making sure that the title and description are adjacent without any space between them.
/* center grid content top-to-bottom */
align-content: center;
align-items: center;
And if I add the following to .outer-grid
, then each of the boxes (.container
sections) have an even height across all rows.
/* makes height even across all rows */
grid-auto-rows: 1fr;