Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial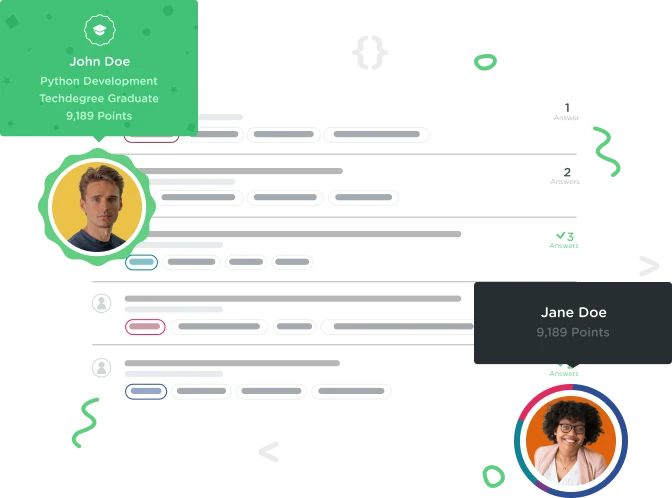

Martin Lynnerup
3,134 PointsHow do I switch on an Enum in Swift, depending on the input I provide?
I have created the below switch statement to return a Double value depending on what string I input in weightType.
I would like to it with an Enum instead, so that it's more flexible. I though it was an easy change but I have tried all kinds of things and I can't make it work.
Can someone please help me? Thx!
var weightType: String = "kettlebell"
func returnWeightLimit() -> Double {
switch weightType {
case "barbell": return(132.0)
case "kettlebell": return(70.5)
case "dumbbell": return(115.0)
default: return(0.0)
}
}
let weightLimit = returnWeightLimit()
3 Answers
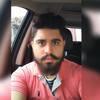
Marlon Burnett
Courses Plus Student 12,917 PointsHey Martin, in that case you could do something like that, i guess...
enum WeightType: String {
case barbell
case kettlebell
case dumbbell
}
extension WeightType {
var weightLimit: Double {
get {
switch(self){
case .barbell: return 132.0
case .kettlebell: return 70.5
case .dumbbell: return 115.0
}
}
}
}
let weightType = WeightType(rawValue: "kettlebell")
if let weightType = weightType {
let weightLimit = weightType.weightLimit
print("Weight limit: \(weightLimit)")
}
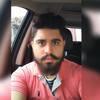
Marlon Burnett
Courses Plus Student 12,917 PointsHi Martin!
I think that would be your best option
enum WeightType {
case barbell
case kettlebell
case dumbbell
}
extension WeightType {
var weightLimit: Double {
get {
switch(self){
case .barbell: return 132.0
case .kettlebell: return 70.5
case .dumbbell: return 115.0
}
}
}
}
let weightType: WeightType = .kettlebell
let weightLimit = weightType.weightLimit
Feel free to reach out for me if you need further help! Best, Marlon

Martin Lynnerup
3,134 PointsHi Marion,
Thanks a lot for the quick answer!
I should probably have mentioned that I still want an input value of type String. That's where it gets tricky I think...

Jeremy Conley
16,657 Pointsenum WeightType {
case Barbell
case KettleBell
case Dumbbell
}
var weightType: WeightType = .KettleBell
func returnWeightLimit() -> Double {
switch weightType {
case .Barbell: return(132.0)
case .KettleBell: return(70.5)
case .Dumbbell: return(115.0)
default: return(0.0)
}
}
let weightLimit = returnWeightLimit()