Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial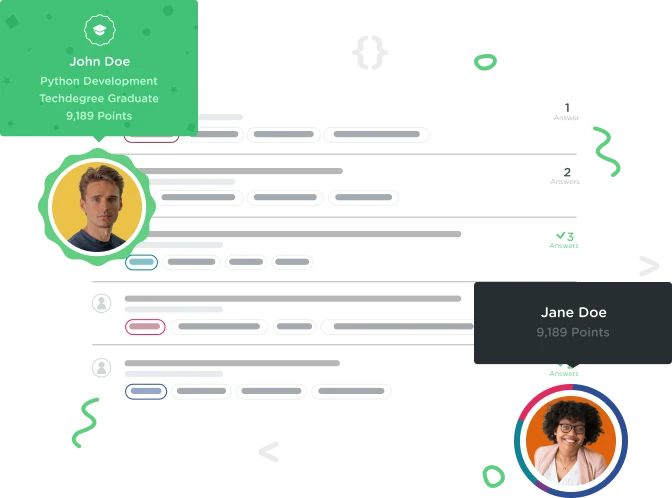
Nyasha Chawanda
7,946 Pointshow do I tackle this challenge
Create a variable named players that is an re.search() or re.match() to capture three groups: last_name, first_name, and score. It should include re.MULTILINE
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search r'(?P<last_name>\w),\s(?Pfirst_name>\w+):\s(?P<score>\dt',string,re.M
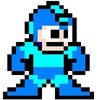
Robert Richey
Courses Plus Student 16,352 PointsIn addition what Gavin said, there are quite a few more issues here. I recommend trying to just capture the last name and then add more to your expression, incrementally testing.
Also, re.search() is a function and needs parentheses. What goes inside are comma-separated arguments to the function.
Here are the Python docs for RE, which I've found to be really helpful.

Gavin Ralston
28,770 Points...and don't forget to review the videos in the treehouse course.
These challenges typically follow very closely what you did in the videos if you were following along. With regex i'd highly recommend taking your time, like RR said, and get bits working at a time. Because regex is really murky territory, no matter who you are. :)
1 Answer
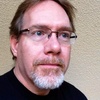
Chris Freeman
Treehouse Moderator 68,423 PointsIn the Players Dictionary and Class Challenge Task 1 of 2
Create a variable named players
that is an re.search()
or re.match()
to capture three groups: last_name
, first_name
, and score
. It should include re.MULTILINE
.
Your current code has a few issues:
players = re.search r'(?P<last_name>\w),\s(?Pfirst_name>\w+):\s(?P<score>\dt',string,re.M
Here are the issues:
-
re.search()
is a method. It's arguments need to be wrapped in parens "( )". - In the sample string, names can contain spaces. Add a space to the matching character set
- Last name needs to match more than a single character. Add plus sign to character set
- Score needs to match more than a single digit. Add plus sign to character set. **For readability I also added brackets "[]"
- <code><</code>first_name<code>></code> parameter is missing the leading "<code><</code>". Add missing "<code><</code>"
- The last named pattern <code><</code>score<code>></code> is missing closing paren ")". Add closing paren.
- There is an extra trailing "t" in the regular expression. Remove trailing "t"
players = re.search(r'(?P<last_name>[\w ]+),\s(?P<first_name>[\w ]+):\s(?P<score>[\d]+)', string, re.M)
The end result: Well done! You're doing great!
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsOne thing I'm seeing is that
(?Pfirst_name>
doesn't match the format of the other two fields you're trying to match. Namely the opening <