Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial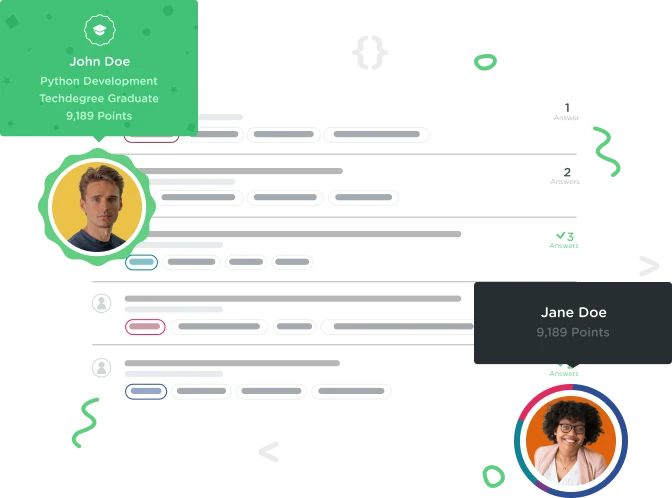

Christian Catalanotto
876 PointsHow do I test to determine which of the two arguments is greater without leaving the function?
I'm not sure how to fit the conditional statement inside of the function, or what that statement should even look like.
function max(1, 2){
}
2 Answers
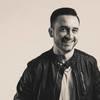
Chris Pulver
7,624 PointsAn if statement looks like this:
if(some condition) {
//do something
}
You can place if statements inside a function block (the two curly braces). It will check the condition once the function is called (e.g. max(input1, input2)).

Matthew Lestina
Courses Plus Student 122 PointsHere is how I would do it:
function max (x, y) {
if (x > y) {
return x;
}
else {
return y;
}
}
Chris Pulver
7,624 PointsChris Pulver
7,624 PointsAlso, just noticed your parameters (1, 2). You should make those non numeric.
Christian Catalanotto
876 PointsChristian Catalanotto
876 PointsThe question is asking me to compare two numbers inside a function and return the larger
Chris Pulver
7,624 PointsChris Pulver
7,624 PointsOk, so first you have your function:
You can name the parameters whatever you'd like, just not a number. Inside the function you have your if statement:
You need to use the same variables so that it references back to the function parameters. If you need to return a value, you use the return keyword:
So your full function would look like this:
To compare two values, you enter your arguments when the function is called:
max(1, 2);
In this example, the program is replacing x with 1 and y with 2:
Since it's frowned upon to give full answers, I'll let you figure out how to return y if it is larger than x. A hint is that right now the function doesn't know what else to do if the first condition is not met.