Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial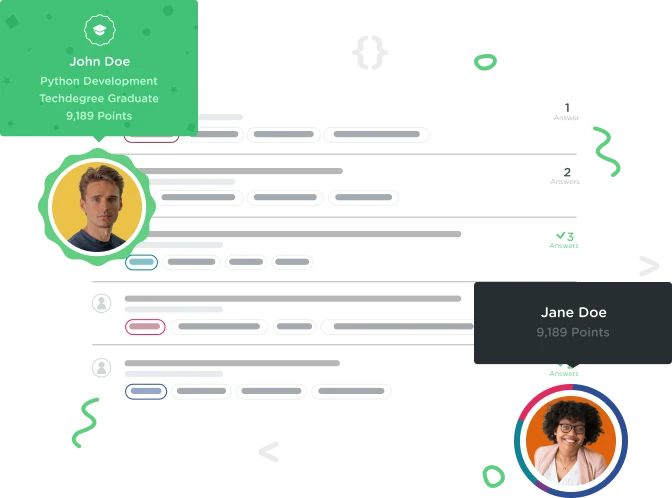

Kenneth Phillips
Courses Plus Student 10,188 PointsHow do I update an integer value with a void method?
I have an app where the user will type the name of an image and if they get it right they will receive a point. I have this process in my checkAnswer method but the value of points will not update except within that method. I have getPoints that retrieves points for another activity where the user can submit the score and it always returns zero. So how would I make it so that points changes all around instead of just in this method?
private Game game;
private ImageView gameImageView;
private TextView gameTextView;
private Button submitButton;
private TextView pointsTextView;
public int points;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game);
gameImageView = (ImageView) findViewById(R.id.gameImageView);
gameTextView = (TextView) findViewById(R.id.gameTextView);
pointsTextView = (TextView) findViewById(R.id.pointsTextView);
submitButton = (Button) findViewById(R.id.submitButton);
game = new Game();
loadPage(0);
checkAnswer(0);
}
private void loadPage(final int pageNumber) {
final Page page = game.getPage(pageNumber);
if (page.isFinalPage()) {
startSubmit();
}
Drawable image = ContextCompat.getDrawable(this, page.getImageId());
gameImageView.setImageDrawable(image);
String pageText = getString(page.getTextId());
gameTextView.setText(pageText);
final int nextPage = page.getNextPage();
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
loadPage(nextPage);
checkAnswer(nextPage);
}
});
}
private void checkAnswer(int pageNumber) {
final Page page = game.getPage(pageNumber);
String gameAnswer = page.getAnswer();
String gameEditText = ((EditText) findViewById(R.id.gameSquare)).getText().toString();
if (gameEditText.equals(gameAnswer)) {
points++;
}
String pointsText = String.format("%1$s", points);
pointsTextView.setText(pointsText);
}
private void startSubmit() {
Intent submitIntent = new Intent(this, SubmitActivity.class);
startActivity(submitIntent);
}
public int getPoints() {
return points;
}
12 Answers
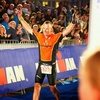
Steve Hunter
57,712 PointsGlad to hear you got it fixed! Good work! Happy to help.
Steve.

Kenneth Phillips
Courses Plus Student 10,188 PointsI will try to get you a version control. I updated the code but it still will not initialize points to the value within the if statement after it checks the answer. This is weird because the pointsTextView does take the updated value and inserts it. It seems to only update it within the method but not outside of it.
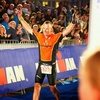
Steve Hunter
57,712 PointsThere are a lot of issues in there. Pushing your code to Github would really help.
Steve.
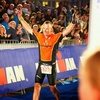
Steve Hunter
57,712 PointsHi Kenneth,
I've not got all the code here - I can't see the getAnswer()
method, for example.
However, you are incrementing points
. It has no value so can't be incremented - you're adding 1 to what?
Try to initialize points
as the activity is created - put points = 0;
inside onCreate()
. That might help.
I think it important to check that gameEditText
holds the value you expect. Looking at what the two variables hold within the if
condition may assist too. That's why I want to see the getAnswer()
method - we can worry about that later.
But keep working on your version control and I'll be able to check all the code that way.
Steve.

Kenneth Phillips
Courses Plus Student 10,188 PointsAlright I believe I've created the repository on Android Studio. I have a vcs.xml file on there but is that the entire project? If not how do I put the entire project on the repository?
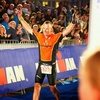
Steve Hunter
57,712 PointsI find that AS is poor at selecting the whole project do I do this manually. You want all your files in there. Let's get the remote sorted first. Decide between Github or Bitbucket. Make the empty remote there and file the instructions about creating the remote connection locally. Then push your local up with git push -u origin master
- we can then see what you put in your local repo!
Steve.

Kenneth Phillips
Courses Plus Student 10,188 PointsSo I've copied the individual important files over including my string.xml file. Will this work or will you need the project as it originally is because my command line is being very difficult pushing the entire project over to my repository?
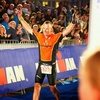
Steve Hunter
57,712 PointsLet me have the link to the repo and I can let you know what I need. Midnight here, so sleep time.

Kenneth Phillips
Courses Plus Student 10,188 PointsNever mind I have got the entire project uploaded. Here is the link: https://github.com/kennethPhillips/newapp.
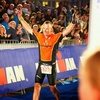
Steve Hunter
57,712 PointsGot that - give me a couple of hours, I'll have a tinker with it at work. I'm sure the boss won't mind.
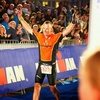
Steve Hunter
57,712 PointsI didn't need to change your code to get the points
variable to increment. I just needed to see what the next value was going to be and pre-empt that! The answer gets out of sync quite quickly. I'm not sure why. I'll keep looking but there's something amiss with the process flow of the game. For starters, clear the EditText once the answer has been submitted - set that value back to a blank string.
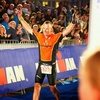
Steve Hunter
57,712 PointsYeah - there's nothing to pause your code to ask for user input. You need to have the code stop. I've added two Toast
s to show you what I mean. I have submitted that as a pull request. You should be able to merge it into your code.
If not, just add these lines:
private void checkAnswer(int pageNumber) {
.
.
Toast.makeText(this, "CODE REACHED checkAnswer", Toast.LENGTH_SHORT).show();
String gameEditText = ((EditText) findViewById(R.id.gameSquare)).getText().toString();
if (gameEditText.equals(gameAnswer)) {
points++;
}
Toast.makeText(this, gameEditText + " " + gameAnswer, Toast.LENGTH_SHORT).show();
.
.
}
This shows you when the code enters checkAnswer
and when it passes beyond your if
statement - it also shows what your gameEditText
variable holds and what gameAnswer
holds too. You'll see how the code doesn't pause so whatever gameEditText
holds from the previous entry is tested against the next picture. If you know the order, you can pre-empt this and enter the next string. When you do that, your points
variable will be incremented.
I hope that helps,
Steve.

Kenneth Phillips
Courses Plus Student 10,188 PointsSo thanks for the help you've already given me. I'm trying to set the textView of the SubmitPage to show many points the user acquired. When I call getPoints() the app crashes though. However the pointsTextView on GameActivity seems fine so how would I set it on the submitPage?
public TextView finalScoreTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_submit);
GameActivity game = new GameActivity();
finalScoreTextView = (TextView) findViewById(R.id.scoreTextView);
String text = String.format("Score: %1$s", game.getPoints());
finalScoreTextView.setText(text);
}
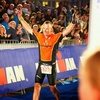
Steve Hunter
57,712 PointsWhat error do you get? And push your code to Github if you've changed it.

Kenneth Phillips
Courses Plus Student 10,188 PointsI haven't changed it . If you run the code you'll see it throws a FileNotFound error almost 20 times, but it already did this and another moderator tell me not to worry about it. Other than that it will not tell me why the app crashes.
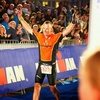
Steve Hunter
57,712 PointsWhen I ran your code I received no such error. I showed you the output I saw.
Talk to me about this error. Which but if code isn't working. What should it so. What does it do.
If your app is crashing, your logcat will have error messages. Try to find those and paste them in here.
But push your code again. Update the remote with your current code. Make sure you do this in your Android terminal:
First:
git add -A
Then:
git commit -m "updated code"
Last:
git push
Sleep for me now!
Steve.

Kenneth Phillips
Courses Plus Student 10,188 PointsI updated it for you but I swear I didn't change anything. If you are not receiving the error maybe it's problem with my computer or this project got corrupted. Let me try to rebuild the project but keep in touch because I might need more help.
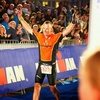
Steve Hunter
57,712 PointsOK - then tell me error you are getting. "The app crashes" isn't explicit. Find out what went wrong in your Logcat - find the red entries and post them.

Kenneth Phillips
Courses Plus Student 10,188 PointsThere is no clear error. I've looked in the logcat and tracked it to the best of my abilities. If you're not getting the same error than I have no idea what is wrong with the application. It only had the FileNotFound errors but I fixed those. So I really don't know what the problem is. I'm going to try to rebuild it and maybe that will work. Do you want me to screenshot my logcat?
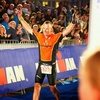
Steve Hunter
57,712 PointsI don't know what actions you are taking in your app to make it do this so it isn't a case of "I'm not seeing it" - I'm just not putting your app in the same position as you.
Let me know what you're doing and I'll see if I get the same error - I will; that's how these things work. And if your app is crashing, there will either be an error, or your code is closing the app.

Kenneth Phillips
Courses Plus Student 10,188 PointsI just probably don't have enough knowledge of android studio to see the problem. The logcat simply says this:
10-18 21:57:32.645 1361-2003/? W/AudioFlinger: power manager service died !!!
10-18 21:57:32.646 1271-1271/? I/ServiceManager: service 'input_method' died
10-18 21:57:32.647 1271-1271/? I/ServiceManager: service 'accessibility' died
10-18 21:57:32.647 1271-1271/? I/ServiceManager: service 'mount' died
10-18 21:57:32.647 1271-1271/? I/ServiceManager: service 'uimode' died
10-18 21:57:32.647 1271-1271/? I/ServiceManager: service 'lock_settings' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'deviceidle' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'device_policy' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'statusbar' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'clipboard' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'network_management' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'textservices' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'network_score' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'netstats' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'wifip2p' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'wifi' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'wifiscanner' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'rttmanager' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'connectivity' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'servicediscovery' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'updatelock' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'notification' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'devicestoragemonitor' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'location' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'country_detector' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'search' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'dropbox' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'wallpaper' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'audio' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'DockObserver' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'midi' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'usb' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'serial' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'jobscheduler' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'backup' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'appwidget' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'voiceinteraction' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'diskstats' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'samplingprofiler' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'commontime_management' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'dreams' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'assetatlas' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'graphicsstats' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'print' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'restrictions' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'media_session' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'media_router' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'trust' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'fingerprint' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'launcherapps' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'media_projection' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'imms' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'user' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'procstats' died
10-18 21:57:32.648 1271-1271/? I/ServiceManager: service 'meminfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'gfxinfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'dbinfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'cpuinfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'permission' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'processinfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'sensorservice' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'battery' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'usagestats' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'webviewupdate' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'scheduling_policy' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'telephony.registry' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'media.camera.proxy' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'account' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'content' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'vibrator' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'consumer_ir' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'alarm' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'window' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'input' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'package' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'activity' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'batterystats' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'appops' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'power' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'display' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'netpolicy' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'phone' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'carrier_config' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'isub' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'simphonebook' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'iphonesubinfo' died
10-18 21:57:32.649 1271-1271/? I/ServiceManager: service 'isms' died
01-01 00:00:00.000 0-0/? E/Internal: device 'emulator-5554' not found
As you can see there are no red errors. The app pretty much just terminates. I'm really not trying to be difficult. I really just don't see what the problem is. I don't know what I'm doing different than you. I'm using the code I sent you. It crashes when I try to load the submitActivity and set the textView of points. When I don't add this specific code it doesn't crash:
public TextView finalScoreTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_submit);
GameActivity game = new GameActivity();
finalScoreTextView = (TextView) findViewById(R.id.scoreTextView);
String text = String.format("Score: %1$s", game.getPoints());
finalScoreTextView.setText(text);
}
I've been talking to another staff member and they can't seem to see the problem either. So that's why I'm wondering if it's a problem with my computer. I'm really trying my best and I'm relatively new to this anyway so I might not have the expertise to see the entire problem.
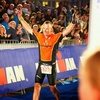
Steve Hunter
57,712 PointsIf you let me know how to get to that part of the game, I'll replicate the error here and get a fix for you. I just need to know how to create the error - what buttons do I need to push. This is not a you thing - it's clearly not about expertise - look how much you've written! This is just one of those bug that, well, bug you! I get them all the time!
So, talk me through the actions I need to take on the device/emulator, and I'll replicate that error and find a fix for you. OK?
Steve.
P.S. I'm not staff - I'm just a guy who helps out on these Community pages. I'm a nobody with a badge! But I'm here to help.
P.P.S. What timezone are you on? I'm in the UK.

Kenneth Phillips
Courses Plus Student 10,188 PointsHey Steve I fixed the problem. My SDK was corrupted which was causing the app to crash randomly. Thanks for all the help and if you submit a comment as an answer I'll mark you best answer. Thanks for helping me to initialize points because that was also causing the app to crash. Other than that the app works great now.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Kenneth,
I can't see where
points
is created? Theif
statement that incrementspoints
lack braces - I don't know how that will affect its outcome.But, first, where is
points
made?Do you have this project in version control so I can pull the entire project and see what's working etc. Try Github or Bitbucket.
Steve.