Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial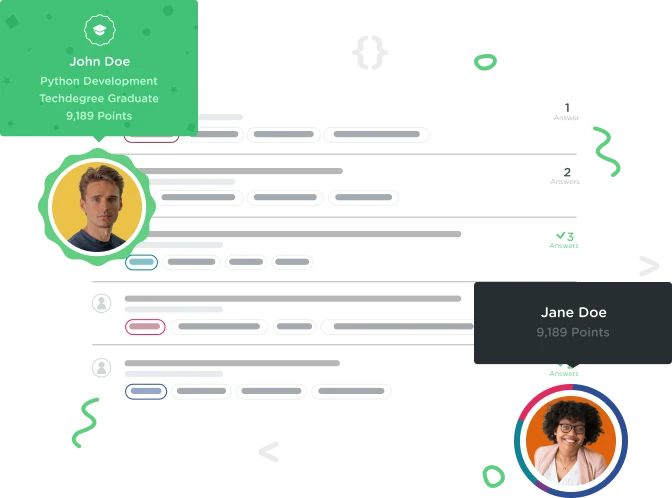
W Joel Baker
1,012 PointsHow do I use an intent with the first FlightActivity challenge?
I'm working on this challenge
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class LaunchActivity extends Activity {
public Button mLaunchButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_launch);
mLaunchButton = (Button)findViewById(R.id.launchButton);
mLaunchButton.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View v) {
private void flightActivity() {
Intent intent = new Intent (this, FlightActivity.class);
startActivity(intent);
}
};
}
}
5 Answers
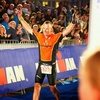
Steve Hunter
57,712 PointsHi Joel,
My code completed this for you. In my head, I didn't use a separate method as you have - that's a question of choice, not correctness.
My onClick()
method was:
public void onClick(View v){
// Add your code in here!
Intent intent = new Intent (LaunchActivity.this, FlightActivity.class);
startActivity(intent);
}
That works for me.
Steve.
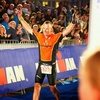
Steve Hunter
57,712 PointsHi Joel,
Again, you're so very close!
An intent starts in the calling class context then moves to the new activity. The first part of this challenge looks pretty similar to what you've already done but it just one line. You don't need to enclose it in a new method.
Intent intent = new Intent(LaunchActivity.this, FlightActivity.class);
Then you need to start it, as you have done:
startActivity(intent);
Hope that helps!
Steve.
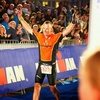
Steve Hunter
57,712 PointsAlternatively, if you just close the method setOnClickListener
by adding a ) before the }; - that might do the trick with your extra method. (I've added a comment in your code to show you!)
W Joel Baker
1,012 PointsHey Steve,
Thanks for helping again! I guess I'm just not clear enough on the structure of developing. Can you show me where/what is the "calling class context" and the "new activity?" Maybe that will help it make sense.
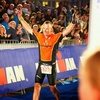
Steve Hunter
57,712 PointsHi Joel,
All this comes in time - the terminology is opaque initially!
The Intent moves from one Activity to another. The 'context' is the current state of the application. The new activity is where you're navigating to.
So, the intent uses both the current state of the app LaunchActivity.this
- sometimes you can just use this
. Then the destination is selected at Class level to point to the right activity (new screen display) to be navigated to.
So, Intent intent = new Intent(context. Activity.class)
creates an Intent called intent, imaginatively. This, when deployed, will change the screen to the Activity coming from the current application state, the context.
Stack Overflow is always a useful site for this sort of thing - sign up to it - a quick Google on there gets you this explanation (no pun intended!!).
Hope that helps! Just keep at it, eventually it slots into place.
Steve.
W Joel Baker
1,012 PointsI just can't seem to put my finger on it. I'll have to pick it back up the morning. But here is where I have left off.
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class LaunchActivity extends Activity {
public Button mLaunchButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_launch);
mLaunchButton = (Button)findViewById(R.id.launchButton);
mLaunchButton.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
// Add your code in here!
flightActivity();}
private void flightActivity () {
Intent intent = new Intent (this, FlightActivity.class);
startActivity(intent);
}
}
});
}
}
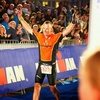
Steve Hunter
57,712 PointsWon't this cause scoping issues? The Intent will only be available inside the flightActivity
method which may be OK but it is worth bearing in mind. The context may need further declaration to bring it back to the class/activity. Again, this is a scoping thing, .this
alone is ambiguous here so it need fleshing out to LaunchActivity.this
.
The individual method is bringing little to the table, here. It creates additional lines of code and isn't something that will be reused elsewhere. I'd ditch that and just call the intent from inside onClick()
as suggested below.
Steve.
W Joel Baker
1,012 PointsThanks Steve! I guess I didn't fully follow you the first time. It may take me longer than most but I will learn it :-)
W Joel Baker
1,012 PointsOH!!!!!!! I added the startActivity(intent); and I wasn't suppose to do that yet in the challenge. Maybe that added bit will help someone else one day.
Thank again for your help Steve! Joel
W Joel Baker
1,012 PointsI had to come back to this before I could go to the next lesson. Trying hard to understand it before moving to the next lesson. I'm getting an error that I'm not understanding (good news is I am starting to get a very small understanding of some of them).
My code...
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_launch);
mLaunchButton = (Button)findViewById(R.id.launchButton);
mLaunchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
startFlight();
}
});
}
private void flightStory (){
Intent intent = new Intent(this, FlightActivity.class);
startActivity(intent);
}
}
The error message is
./LaunchActivity.java:20: error: cannot find symbol
startFlight();
^
symbol: method startFlight()
1 error
W Joel Baker
1,012 PointsOh, I did try (LaunchActivity.this, FlightActivity.class); but that produced another error.
Found the an error startStory();. fixed it to startFlight(); but still didn't get rid of the error.
YES!!!!!! I got it. Typo typo typo.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_launch);
mLaunchButton = (Button)findViewById(R.id.launchButton);
mLaunchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
startFlight();
}
});
}
private void startFlight (){
Intent intent = new Intent(this, FlightActivity.class);
startActivity(intent);
}
}
W Joel Baker
1,012 PointsW Joel Baker
1,012 PointsI'm getting closer... But still need help.
//The compiler errors are