Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial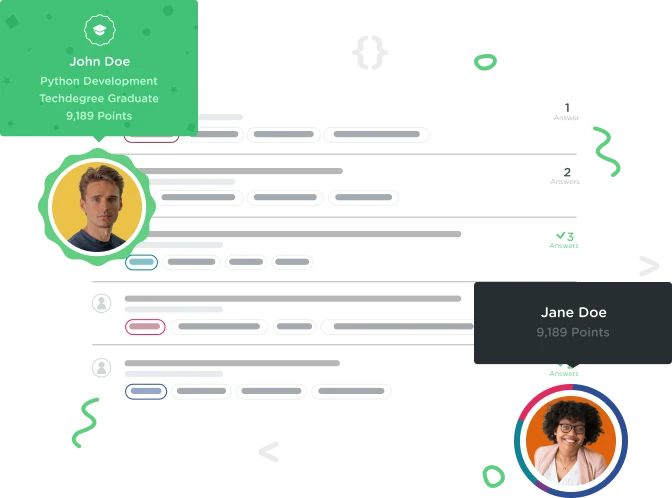

Abe Daniels
Courses Plus Student 2,781 PointsHow do I write a constructor that will eliminate the possibility of passing string data that contains a symbol?
I am running into an issue where the user is allowed to enter an underscore and they should not be able to. The issue is, how do i know if just an underscore will be enough? That makes me think that I need to write some sort of rule that instead of eliminating the use of characters I would be restricting the user to using letters only. How should I implement this?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char mChar = 'm';
if (fieldName.charAt(0) != mChar) {
throw new IllegalArgumentException("Your must start your field with an 'm.'");
}
if(Character.isLowerCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("The second letter must be uppercase !");
}
return fieldName;
}
}
3 Answers

Dan Johnson
40,533 PointsLuckily you don't have to implement all the logic yourself, the Character
class has static methods dealing with this sort of thing. You can leverage isLetter in order to determine the type of character and use that to determine if you should throw an exception or not.
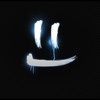
Grigorij Schleifer
10,365 PointsBut where i define which letter it must be ??? For example, i dont wont a 'z' ....
I am a little confused

Dan Johnson
40,533 PointsIf you're wondering how isLetter classifies what a letter is, and what isn't, It considered the following Unicode categories:
- OTHER_LETTER [Lo]
- MODIFIER_LETTER [Lm]
- TITLECASE_LETTER [Lt]
- LOWERCASE_LETTER [Ll]
- UPPERCASE_LETTER [Lu]
You can select the "list" link in the bottom left to see all the characters, though some like Lo have over 13,000 so expect long load times.
If you meant how to check for something like z, you can just use the same method as above, but compare against the character literal ('z'
in this case).
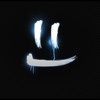
Grigorij Schleifer
10,365 PointsSo te word "letter" is "preinstalled" in the Character class and contains a lot of different chars ... ?

Dan Johnson
40,533 PointsBasically the isLetter method uses the Unicode definitions (which are predefined in the context of the program) to check the character.
As for how isLetter works exactly, I couldn't tell you. If the characters are all in a well defined range it could simply check if it fell in those ranges, but that's just an idea.
Abe Daniels
Courses Plus Student 2,781 PointsAbe Daniels
Courses Plus Student 2,781 PointsI did happen to come across this once i thought about it further. But how would I check for every letter in the string while still remaining DRY?
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsUsing a for in loop is one solution if you want to check every letter: