Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial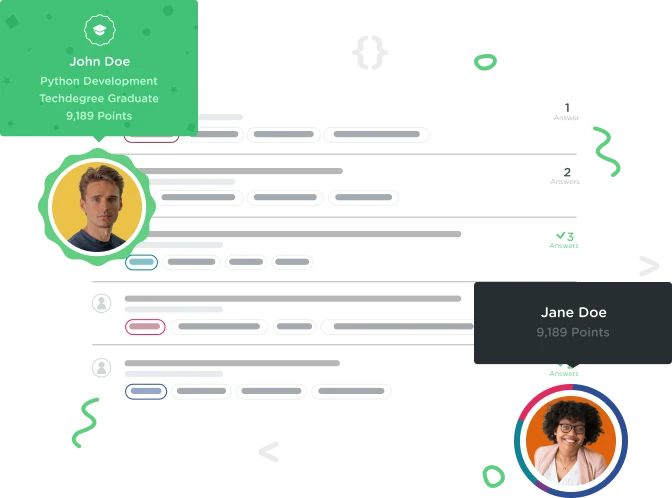
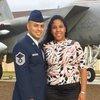
Jonathan Castro
493 PointsHow do I write a program that collects 3 ints from a user using a WHILE loop, maintain a running total, & print the sum?
I want to write a Python program that collects three integers from the user using a WHILE loop, maintain a running total and report the sum after three values have been totaled.
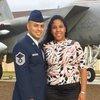
Jonathan Castro
493 PointsIt's got to be in a WHILE loop in order to get the question correct. This is for my Python programming college class. I was able to build the same thing with a FOR loop, but can't figure out how to do the same for a WHILE loop. Here's what I got for the FOR:
total = 0 for i in range (3): sum_of_numbers = int (raw_input("Enter any number: ")) total += sum_of_numbers print "\nThe sum of these numbers is:", total
How can I get the same result using WHILE?
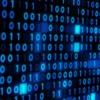
Alexander Davison
65,469 Pointstotal = 0
for i in range (3):
sum_of_numbers = int(raw_input("Enter any number: "))
total += sum_of_numbers
print "\nThe sum of these numbers is:", total
Hmm, so you are using Python 2. Ok then. I can't write in Python 3 then XD
total = 0
i = 0
while i < 3:
sum_of_numbers = int(raw_input("Enter any number: "))
total += sum_of_numbers
print "\nThe sum of these numbers is:", total
i += 1
Hope it helps!
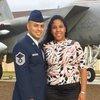
Jonathan Castro
493 PointsHow do I get it to print ("\nThe sum of these numbers is:", total) only at the end of the program? Right now, it prints this prompt after each user input. Sorry for being so picky. The professor wants it to return like this:
Enter any number: 1 Enter any number: 2 Enter any number: 3
The sum of these numbers is: 6
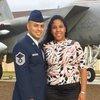
Jonathan Castro
493 PointsWoo, hoooooo!!! That's it! Thank you!!!
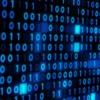
Alexander Davison
65,469 Points:D I am happy you finished you task!
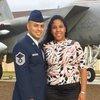
Jonathan Castro
493 PointsThanks Xela! You don't know how long I've been racking my brain on this exercise! The crazy thing is, the code wasn't that far off from the FOR loop! Lol!
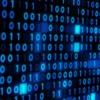
Alexander Davison
65,469 PointsXDDDD I know, right?
1 Answer
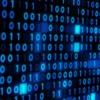
Alexander Davison
65,469 PointsAll right. That is actually VERY easy to change. Try this:
total = 0
i = 0
while i < 3:
sum_of_numbers = int(raw_input("Enter any number: "))
total += sum_of_numbers
i += 1
print "\nThe sum of these numbers is:", total
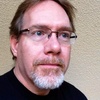
Chris Freeman
Treehouse Moderator 68,423 PointsMoved to answer.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsSo I am guessing you want to know how to ask for three inputs, and print the sum of them? If so, I would do this:
Hope that helps! If this does not work, please tell me.