Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial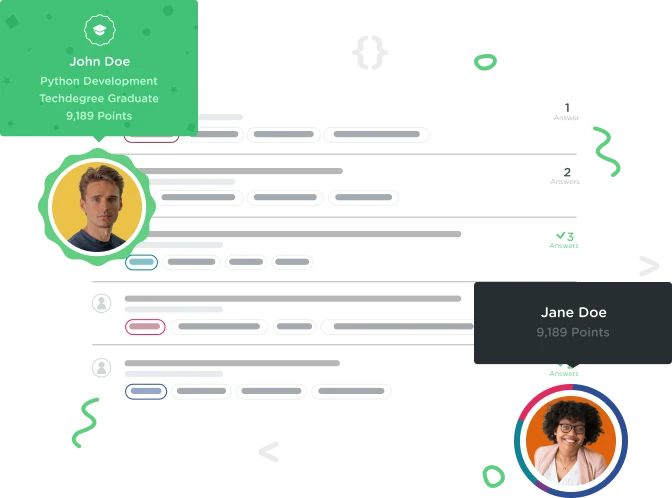
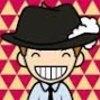
Aaron Selonke
10,323 PointsHow do Interfaces work?
In C#, for example, a List<T> inherits from the IEnumerable<T> which is an interface.
An Interface by definition only has method signatures (empty methods with no programming logic).
Classes that are derived from these Interfaces are supposed to 'implement' these Interfaces by using all the method signatures supplied by the interface - but the logic of each of these methods should be provided in the derived class?
Does this sound correct?
Following my example of the List<T> and the IEnumerable<T> I'm trying to understand 1) Why are we able to instantiate an IEnumerable<T>, Isn't it just an Interface? 2) Why are there specific definitions in the MSDN for the methods of IEnumberable<T>. Because its only an interface shouldn't these just be Method signatures?
4 Answers

William Schultz
2,926 PointsAaron, If you look at the classes that implement ISet<T>, you will see that they have the methods within the class. If you were to create a class that implements ISet<T> then inside your class you would have to create each method listed in the interface and provide the logic of each method.
It seems counter intuitive at first; I know it did for me. But from my current understanding of interfaces, the real power comes from the fact that you can refer to the interface in your code instead of the classes that implement them. This allows you to, say for example, use a foreach loop on items of type IEnumerable instead of type List and type ArrayList. The foreach loop won't care that it is a List or an ArrayList, because both have implemented IEnumerable, i.e. are of type IEnumerable to the compiler. So instead of having to run multiple loops for each type, List and ArrayList, it all can be done in one loop. (I think this is a bad example on my part as I am basically talking about a list of a collections, which is confusing. Sorry.)
Another example:
I create an interface named IAnimal and require any class that implements it to have a Move method.
I then create a class named Dog and implement the interface. This means I have to write a Move method inside my Dog class and provide the logic for the Move method.
I also create a class named Turtle and implement the IAnimal interface. Again I have to implement the Move method inside my Turtle class. (a Dog may move differently than a Turtle.)
Anyway, Say I want to iterate through an ArrayList holding objects of both Dog and Turtle. I can use a foreach loop and iterate through the ArrayList of 'IAnimal'. If I just used 'Dog' then it would error out and not understand when it pulled a Turtle from the ArrayList because you told it the ArrayList had Dogs in it and a Turtle is not a Dog. But both Dog and Turtle ARE IAnimals as they both implement the required method from the interface.
This also allows you to make changes to your Dog and Turtle classes without affecting the code that implements the use of IAnimal, like where your foreach loop is. You can change Dog and add methods and properties and it would not affect the previously written code. This also allows for extensible code as later you can add a 'Cat' class that implements IAnimal and all of your previous code would work fine with any 'Cat' objects too.
This is my current understanding of Interfaces. If I am incorrect in anything I have said, please correct me if you are more knowledgeable. Hope this helps explain it a bit better.

Samuel Glover
1,932 PointsHi Aaron,
Reading through your first two paragraphs, it sounds like you have indeed grasped the concept of interfaces vs their implementations. Everything you are saying is correct so far as I can tell.
To answer your questions:
1) You should not be able to instantiate an interface. Attempting to do the following should give you a compiler error:
IEnumerable<string> myListOfStrings = new IEnumerable<string>();
What you may be referring to is the following code:
IEnumerable<string> myListOfStrings = new List<string>();
The reason this line will compile is because the type List<string> implements IEnumerable<string>. In fact, List<string> is far more powerful than an IEnumerable<string> but the key point is that it has AT LEAST the functionality defined by the IEnumerable<T> interface and therefore is a valid implementation of that interface.
2) I don't fully understand your question but I will try my best to answer. The reason MSDN describes all of the features of IEnumerable<T> is to inform developers that, so long as they are working with an IEnumerable<T> implementation, they can expect to be able to make use of any of the methods described in the documentation.
Hope this helps a little.
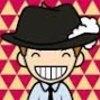
Aaron Selonke
10,323 PointsThank you, It is a really good explanation.
To clarify about the MSDN's interface definition.
Here is a screenshot of the MSDN's definition for the ISet<T> interface.
http://uploadpie.com/hUXlt
Here there is a list of Definitions for the properties, methods, and extension methods for this interface.
There first method is ISet<T>.Add Method (T).
Screenshot here:
http://uploadpie.com/NdNSD
I'm trying to understand why there are methods with definitions for this interface?
I would understand this fully if it was a class, but interfaces (as far as I understand) do not have method's with bodies. How, for example, should we be able to implement the ISet<T>.Add method.
As an interface, this method should only be a method signature, and not a fully defined method. How do we get to use all of these useful methods just by implementing an interface, where is the code/logic for these methods? They are not in the interface, because the interface merely has a method signature.
It looks like the MDSN treats the documentation of Interfaces the same as Classes...
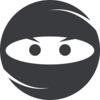
John Lundberg
20,368 PointsHis original question was never answered here and i am actually wondering the same.
A interface doesnt have any logic for their methods in them(They are interfaceses so thats the point). How come an interface can have methods with logic inside them such as ICollection<T>.Add()?
Does the interface inherit the logic or is there some kind of double standard going on?

Adam McGrade
26,333 PointsIf I understand correctly, the List<T> implements the ICollection<T> Interface.
The ICollection<T>.Add() method implementation would be provided on the List class. There is no actual logic for the Add method on the ICollection interface.
After doing some research, I found the actual implementation of List class in the .NET source code. It can be seen here http://referencesource.microsoft.com/#mscorlib/system/collections/generic/list.cs,cf7f4095e4de7646
You can see in the source code how the List class implements the ICollection interface.
Hopefully that clears things up for you, it made a lot more sense to me once I read through the source.
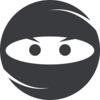
John Lundberg
20,368 PointsThis explanation makes sens! Thank you Adam MacGrade!
chanjong kim
2,315 Pointschanjong kim
2,315 Pointsfantastic explanation. thanks .