Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial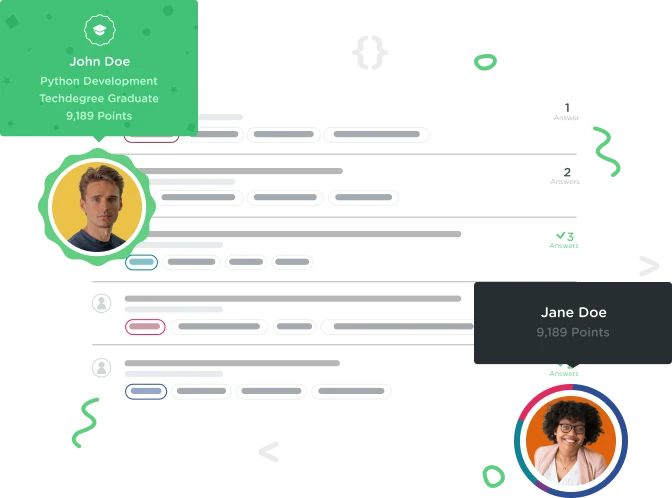

Aditya Gawade
1,182 Pointshow do proceed? I'm stuck at cause I'm not able to apply. what concept am i lacking? please help
In the editor, I’ve declared a struct named RGBColor that models a color object in the RGB space.
Your task is to write a custom initializer method for the object. Using the initializer assign values to the first four properties. Using the values assigned to those properties create a value for the description property that is a string representation of the color object.
For example, given the values 86.0 for red, 191.0 for green, 131.0 for blue and 1.0 for alpha, each of the stored properties should hold these values and the description property should look like this:
"red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0"
Note: Init methods typically list parameters in the same order of property declaration. For this task, stick to the order red,green,blue,alpha.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
let description = return "red:"
// Add your code below
}
3 Answers
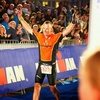
Steve Hunter
57,712 PointsHI Aditya,
Have a read through this thread which might help you out.
A couple of things. First, you don't want to hard-code the number values; they are what get passed into the init
method. So, self.red = 86.0
should be self.red = red
- the second red
is passed in by the user; they want to be able to create a struct containing any color, right?
Then, inside the init
method, build up the description
string, as in the question, using string interpolation. Something like self.description =
"red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)
".
I hope that helps,
Steve.
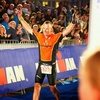
Steve Hunter
57,712 PointsHi Aditya,
The init
method is used to make sure all stored properties are given a value as the instance of the struct is created.
Each property can be set inside init
directly, or the uer can pass a value in as the instance is created. Let's look at the directly set way first. This is what you set off doing in your code at the beginning of this thread. Each value for red, green etc. is set inside the init
method.
init() {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
In this way, the init
method takes no parameters; it doesn't need them as it will do nothing with them. All stored properties are set wthin the init
method, so the compiler is happy. BUT every struct is identical and cannot be changed. Obviously, this is not what we want.
So let's look at how else this can be done. This example is the answer to the challenge. The challenge wants a user to be able to create a struct to represent any color. While there are no bounds-checks or tests in this example, let's assume that the user won't pass in a number outside the range 0 - 255, as that's what the RGBA representation is trying to achieve.
The color representation is determined by the four constituents, RGB & A. The description
stored property is made up of a string with the other four properties' values interpolated into it. The user doesn't need to present any additional information to construct the string correctly - so the user only needs to provide the RGB & A values. So, the init
method now takes four parameters; red
, green
, blue
and alpha
but the struct has five stored properties. THis is fine, as long as the init
method sets all five properties to contain a value, then the compiler is happy. The init
method has enough information to set all five values even though only four are being passed in - the fifth is made up of the other four and no other information:
init (red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
The compiler wants to have all five properties set using the init
method; that' done, so it is happy. The user wants to be able to create a representaion of any color by passing in chosen values for red
, green
, blue
and alpha
; that's done so the user is happy too. A color representation can be created like:
let someColor = RGBColor(red: 21.2, green: 32.3, blue: 43.5, alpha: 65.7)
The struct will be created, inserting thoe values in to each of the red
, green
, blue
and alpha
stored properties. The description
will look like:
"red: 21.2, green: 32.3, blue: 43.5, alpha: 65.7"
I hope that helps explain why an init
method is used and why is in't necessary to pass every stored property into the init
method. The init
method must set a value for all stored properties but those properties can either be hard coded or made up of other parameters. So, in the above example, there is no need for the user to pass in a string for the description
propery as it could be correctly created without any user-input.
Steve.

Aditya Gawade
1,182 Pointsthank you s o much for ur time and answer. it really helped!
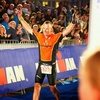
Steve Hunter
57,712 PointsNo problem!

Aditya Gawade
1,182 Pointsthank you very much steve but that thread gave me a doubt which i did not realise myself and now I'm a bit confused; why do we use init and why did we not put description in init?