Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial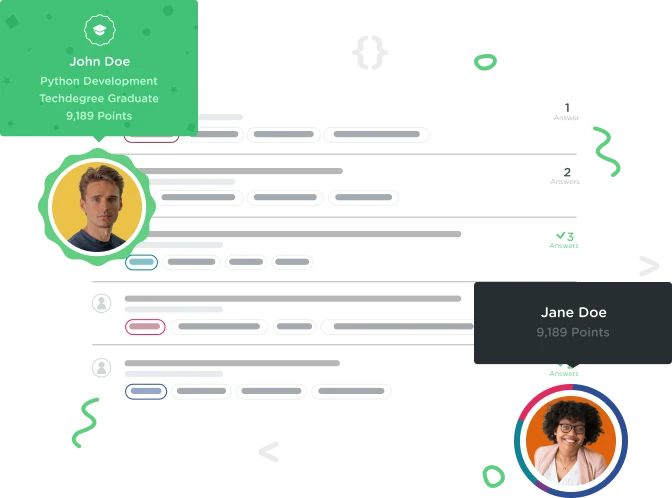

Moses Ong
165 PointsHow do tuples hold two ordered variable
I kept getting syntax errors. I wonder how I should work around with the solution
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(string, string1):
string = list(enumerate(string)
string2 = list(enumerate(string2)
joined_string2 = string + string2
return joined_string2
1 Answer
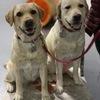
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHI Moses,
def combo(string, string1):
First, I'd strongly recommend using better names for your parameters. Your function definition suggests to anyone reading it that this function only works with, or only expects to receive, strings as inputs. This is false. You will get all kinds of iterables as inputs. So perhaps naming your parameters: iter1
and iter2
would make your code more clear.
string = list(enumerate(string)
string2 = list(enumerate(string2)
Having argument names that reflect that type of data you are receiving will help you to remember that you don't need to turn your inputs into lists. The code to solve the challenge will work directly on any kind of iterable. Also,
you called your second input string1
in your function definition but you're referring to it as string2
here.
joined_string2 = string + string2
Let's take a look at the Python interpreter and see what this would produce, using the sample inputs [1, 2, 3], 'abc'
:
[(0, 1), (1, 2), (2, 3), (0, 'a'), (1, 'b'), (2, 'c')]
And this is what the challenge wants as an output:
[(1, 'a'), (2, 'b'), (3, 'c')]
As you can see, you've added the 0,1,2
sequences to both iterables (using the enumerate
function). Also, your sequence is all of string
then all of string1
but the challenge wants you to combine the elements so you have the both the first items, then both the second items, etc.
The easiest way to do that (where by easy I mean the easiest code concepts we've seen before) would be to:
- create an empty list, then make a loop that iterates the number of times of the length of one of your iterators (remember that the challenge says they are both the same length).
- During each iteration of the loop, it makes a tuple of that index's element from iterator 1 and that index's element from iterator 2 and then
- Append each tuple to your list; finally
- return the list
There is also a really cool function called zip
that will basically do all the above in one line, but it's probably best to really cement your understanding of all the above before you add that into the mix.
Cheers
Alex