Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial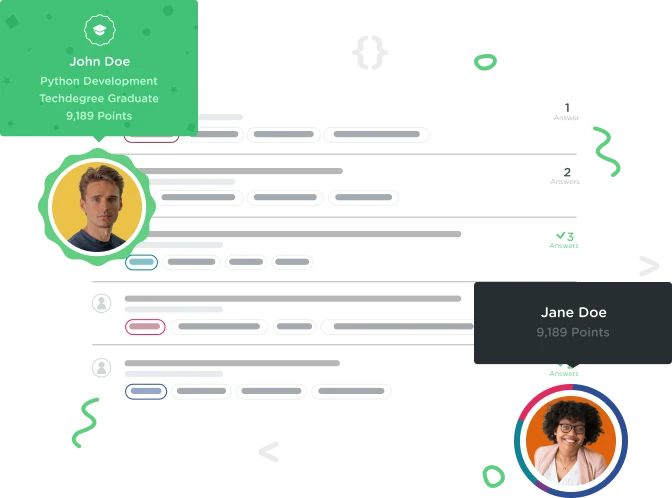

Kharl McCatty
3,072 PointsHow do we add a custom background picture to every knew fact instead of a new color
I was doing the tutorial on how to add custom background colors after every knew fact in the creation of the fact finder app. Does anyone know how we can add our own custom background picture to every knew fact?
i used this format to change the color of one of the backgrounds
relativelayout.setBackgroundColor(Color.RED);
is there a way to use a similar format to change the background picture?
2 Answers
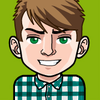
Harry James
14,780 PointsYeah! Here's a quick way to do this:
In FactBook.java, create this member variable:
protected static int randomNumber;
Then, make this change in the same file:
// (-) Delete this line: int fact = mFacts[randomNumber]; Replace with this \/
fact = mFacts[randomNumber];
Now, you've made the randomNumber variable accessible from anywhere within your package!
Next up, in ColorWheel.java, make this change:
// (-) Delete this line: color = mColors[randomNumber]; Replace with this \/
color = mColors[FactBook.randomNumber];
Now, you're using the randomNumber from FactBook.java so, if 2 was called, for example, then the fact at index 2 and color at index 2 will be displayed! Woohoo!
You do also have some spare code so, you can also get rid of this to improve performance:
// (-) Delete this line: Random randomGenerator = new Random();
// (-) Delete this line: int randomNumber = randomGenerator.nextInt(mColors.length);
Hope it helps and, if you have any more questions, give me a shout :)
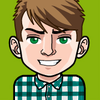
Harry James
14,780 PointsOh I'm sorry! I misread your question.
Yes, use an ImageView instead and follow the same technique as above but use a custom URI instead of a color :)

Kharl McCatty
3,072 PointsThank you. Just a few questions to clear it up because i'm still really new to android. Would I create a URI for @drawable/(name_of_image) and then place the URI's in an array and then assign them using image View
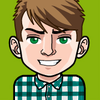
Harry James
14,780 PointsI'll go through how you do this for you :)
First of all, in res/values, create a new arrays.xml file and paste this as the contents:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<array name="factImages">
<item>@drawable/image1</item>
<item>@drawable/image2</item>
<item>@drawable/image3</item>
</array>
</resources>
This will create a new TypedArray in XML.
Then, in code, you can access these images like this:
Resources res = getResources();
TypedArray factImages = res.obtainTypedArray(R.array.factImages);
Drawable factImage = factImages.getDrawable(0);
I'll leave the final bits to you as a challenge but, if you have any problems with this, I'll happily tell you how to do it :)
Kharl McCatty
3,072 PointsKharl McCatty
3,072 PointsThank you for your answer however you didn't answer the question, your response answers how to add color but is there any way to add a background picture instead of a background color.