Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial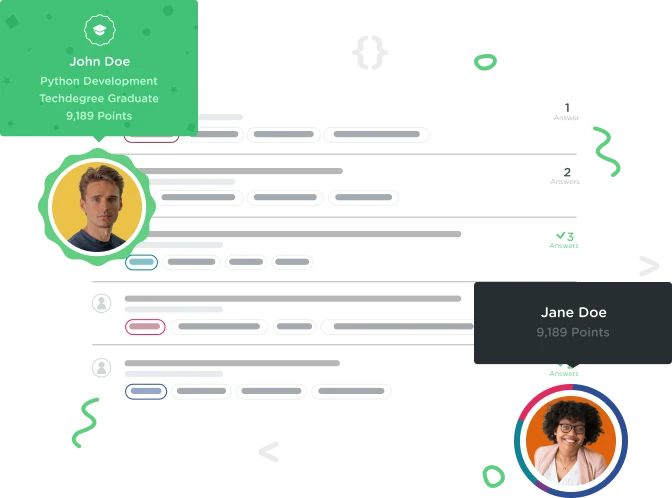
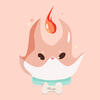
kalyada leosrisook
Courses Plus Student 17,855 PointsHow do we fix the " is not abstract and does not override abstract method compareTo(Object)" error?
hi, I was trying to override the compareTo method in java, I added the implements word on the class title and put the @Override sign above the method I want to override and I got the " is not abstract and does not override abstract method compareTo(Object)" error message. I want to know how to fix this. Could anyone here help me out ? thanks!
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
@Override
public String compareTo (Object obj)
{
//trying to make it return 1...
obj = 1;
obj2 = 0;
result = obj.compareTo(obj2);
return result;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
1 Answer

andren
28,558 PointsWhen you get compiler errors it's worth taking a look at all of them and not just the first that pops up if that is not helpful, the second error in the compiler for your code is this:
./com/example/BlogPost.java:45: error: compareTo(Object) in BlogPost cannot implement compareTo(T) in Comparable
public String compareTo (Object obj)
^
return type String is not compatible with int
where T is a type-variable:
T extends Object declared in interface Comparable
That errors message contains a lot of complicated stuff, but one line in particular is actually pretty straight forward, namely return type String is not compatible with int
. This is an error message that points out that your method has a return type of String while the method you are trying to override has a return type of int.
That is the main issue with your code, the method has the wrong return type, as you are not returning a String but an int. It's also worth noting though that you use two variables in your method (obj2
and result
) that are not actually declared anywhere, which means that they would have produced an error as well.
In order to return 1 you don't need to do anything beyond literally returning 1 like this:
@Override
public int compareTo (Object obj)
{
return 1;
}