Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial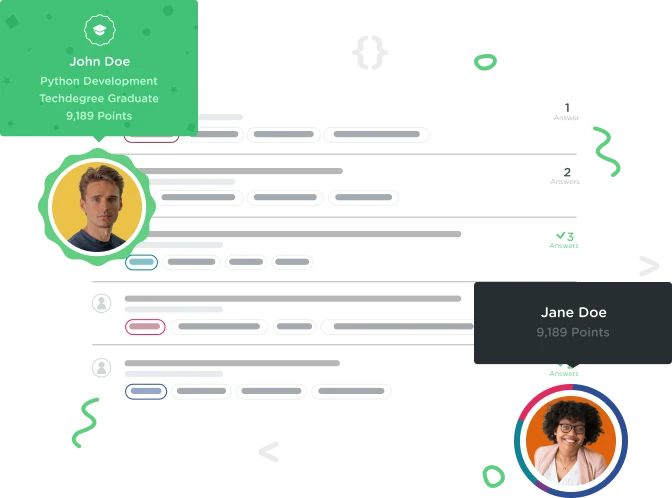

Maneesh Kumar
Courses Plus Student 321 PointsHow do we print out just the decimal part of a float value?
Eg. I want to print out .15 in 273.15 .
3 Answers
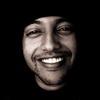
miikis
44,957 PointsHey Maneesh,
The easiest, and most practical, method to accomplish this task is the modulo operator. It's rendered as the percentage sign (%) and it works like this.
var num1 = 100, num2 = 7;
var answer = 100 / 7; // returns 14.28571429
var remainder = 100 % 7; // returns 2
So, if you're just trying to get the last 2 digits of a float, you could use some String method (like slice). But more than likely, you're trying to find the remainder... and that's what the modulo operator does. Let me know if that answers your question.
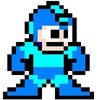
Robert Richey
Courses Plus Student 16,352 PointsHi Maneesh,
// note: this is a number and not a string
var float = 273.15;
// let's get the position of the radix-point
var radixPos = String(float).indexOf('.');
// now we can use slice, on a String, to get '.15'
var value = String(float).slice(radixPos);
console.log(value); // '.15'
Cheers

Maneesh Kumar
Courses Plus Student 321 PointsThanks for the help bud but this stuff just flew over my head.
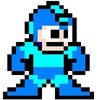
Robert Richey
Courses Plus Student 16,352 PointsHmm, for now feel free to use the function below. Call the function with any number you want to extract the mantissa - including the radix point - which is called a decimal point when values are base 10.
I do apologize if it seems like I'm over-complicating this - I'm not trying to. I'll be more than happy to try and answer any specific questions you may have. If none of this makes sense, I wouldn't know where to begin.
function getFraction(n) {
var s = String(n);
return s.slice(s.indexOf('.'));
}
// example usage
var number = 273.15;
console.log(getFraction(number)); // output: '.15'
// another example
var pi = 3.14159265359;
console.log(getFraction(pi)); // output: '.14159265359'
All the best,
Robert

Maneesh Kumar
Courses Plus Student 321 PointsOK got this one, thanks for the help.
Maneesh Kumar
Courses Plus Student 321 PointsManeesh Kumar
Courses Plus Student 321 PointsFirst of all thanks for answering and abiut the answer, i am looking for a way to seperate the decimal part from the whole number part.Like in your answer above i want the .2857... part separated from the returned answer so it reads something like 100/7 = 14 + .2857....
miikis
44,957 Pointsmiikis
44,957 PointsGotcha. Well, take a look at this then:
Is that more helpful?
Maneesh Kumar
Courses Plus Student 321 PointsManeesh Kumar
Courses Plus Student 321 PointsSure is, thanks Mr. Woodwinter.