Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial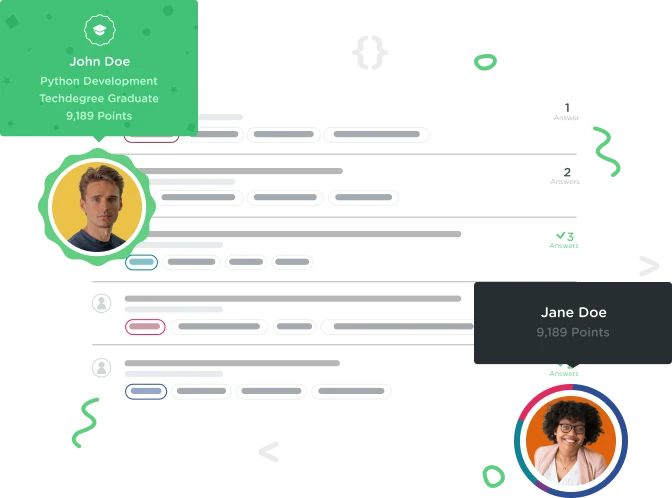

Maxwell Catmur
7,355 PointsHow do you add integers of arrays together?
Here is the question:
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
Here is the code I have typed. Please can you let me know what I've done wrong?
let numbers = [2,8,1,16,4,3,9] var sum = 0 var counter = 0
while counter < numbers.count { sum += numbers[] counter += 1 }
Thanks
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum += numbers[]
counter += 1
}
2 Answers
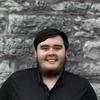
Michael Hulet
47,912 PointsYou can't just add all the elements of an array together like that, though it'd be cool. Using a for
loop instead of a while
loop with a counter would be right up this question's alley
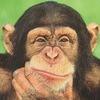
mrtroy
8,069 PointsIt looks like you forgot to use the index value for numbers inside the while loop. As you go through the loop counter will start as zero and end up with the number or items in "numbers[]" minus one. In this case counter will go from zero to six.
You can test that by putting a print(counter) inside the loop. Try it just before the sum is added.
Once you have done that change the print to be "print(numbers[counter])" and you can see each element in the array. You should be able to see what to do next to fix that sum addition statement.
Happy coding!
PS. I know you got your answer a few hours ago but I still wanted to give you some troubleshooting tips. Put some print statements in a loop to see what it is doing.

Maxwell Catmur
7,355 PointsThank you very much
Maxwell Catmur
7,355 PointsMaxwell Catmur
7,355 PointsThank you. But this question is specifically requesting for me to use a while loop.
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsOhh lol that's what I get for just answering without looking at the question :P In that case, your code looks mostly good, but to get a value out of an array, you need to provide a number to its subscript. For example
numbers[0]
gets the first value in thenumbers
array. What variable in your code starts at0
and increases by1
for as many objects as there are in thenumbers
array?Maxwell Catmur
7,355 PointsMaxwell Catmur
7,355 PointsThank you very much Michael; it works! :D