Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial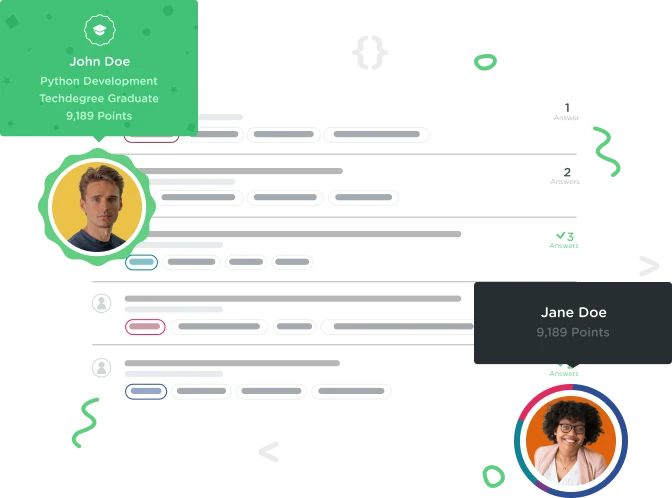

Anthony Lopez
963 PointsHow do you add values from a for loop?
Hey guys!
I have two questions for this challenge.
Is there a way to take out values from an argument aside from using a loop if the argument is unknown?
Is it possible to add the values that come out of a for loop?
My struggle so far is that I need to take the values from a dictionary that gets put into my courses function and combine them into a single list. I can get the values, but all my brain keeps thinking is that I need to add those values together since they are two separate lists.
However since each list is tied to the course variable in the for loop I can't add course + course. All that gets me is a doubled version of each list.
Thanks! Appreciate the support that exists here!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(sing_arg):
return len(sing_arg)
def num_courses(sing_arg):
value = 0
for courses in sing_arg.values():
for answer in courses:
if answer in courses:
value += 1
return value
def courses(sing_arg):
for course in sing_arg.values():
1 Answer

Kirsten Smith
3,484 PointsWithin your function argument and before you begin the loop, I suggest making an empty list, so new_list = [] or something of that nature. Within the for loop, then you can perform your check, so if a certain criteria is met, then you add that object to the new_list (if block). This is easier than trying to alter the list that is passed in.
Anthony Lopez
963 PointsAnthony Lopez
963 PointsHey Kristen thanks for the suggestion!
I actually thought of that one, but won't I run into the same problem? How do I add the values once they are in the list, because while they are in the function they are tied to the for loop variable course.
Also won't I end up with a list within a list?
Thanks!
Kirsten Smith
3,484 PointsKirsten Smith
3,484 PointsAnthony, when you are within a for-loop, you aren't within a list. Basically, all you are doing is setting what you want to do for EACH ITEM within a certain list. That does not mean that you are physically within the list during execution.
Take this example code:
When you run this, you simply get back a list of the first character in each item of the list items. Does this make sense?
Anthony Lopez
963 PointsAnthony Lopez
963 PointsHey Kristen that's a good suggestion. Here is my dilemma though:
Example argument for the challenge {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
As you can see the values within the dictionary are lists. That's why I mention that using that method I would get a list within a list.
If I were to use new_list.append(course[0]) it would put just jQuery Basics and Python Basics into the new list, but I need Node.js Basics and Python Collections as well.
If I were to try to use new_list.append(course[0:]) to try to grab everything within the list, it just returns the entire list and I end up getting a list within a list like I said. new_list = [['jQuery Basics', 'Node.js Basics'], ['Python Basics', 'Python Collections']] The challenge will not accept this.
If I were to try to use a series of appends like new_list.append(course[0]) new_list.append(course[1]) it would not work because I will not know how many courses the challenge decides to put in the list when calling the argument. It could be 3, 4, or 5 courses within the list. When I tried it said I did not get all of the courses in the list.
What do you think?
Kirsten Smith
3,484 PointsKirsten Smith
3,484 PointsI understand your question. There is actually a function designed specifically for this application; list.extend(list_adding). This will essentially add each ELEMENT of a list to your list, in this case, your new_list. So if you try the code with just extend instead of append it will eliminate the problem with having a list inside of a list. I apologize I didn't realize that was what you were asking initially, hope this helps.
Anthony Lopez
963 PointsAnthony Lopez
963 PointsThanks Kristen! I can't believe I forgot that one.
It's definitely in my brain but for some reason it did not come to the front haha.
Appreciate your help a lot!