Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial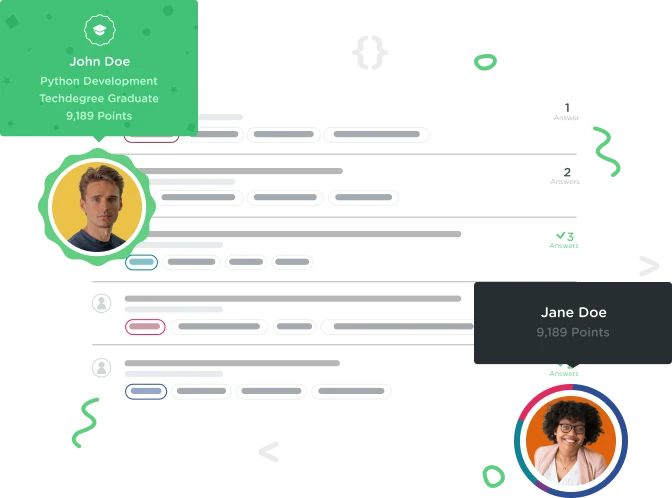
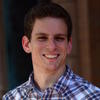
Jeff Lange
8,788 PointsHow do you call a method from inside another method? Should I be using some sort of loop?
I'm a ruby newb, and I like to invent simple, personal challenge programs for myself. Today my task was to create a program that would add two numbers inputted by the user.
I got the basic program to work fine. The issue I'm having is that I want to give the user the choice of having the program restart. Unlike in javascript, it appears I can't call a method ("function" in javascript terms) from within another method. The code below yields the error:
"rb:29:in 'again': undefined local variable or method 'add_num' for [object ID] NameError)."
I've tried getting for and while loops to work, but I'm obviously not grasping something enough to make it happen. Here's the code:
class Adder
def initialize ()
end
def add
puts "This app will add two numbers together for you! Please select your first number. "
x = gets.to_f
puts "Excellent! Please select your second number. "
y = gets.to_f
puts "The sum of your numbers is #{x + y}. "
end
def again
puts "Would you like to add again? (y/n)"
response = gets.chomp
if response == "y"
add_num.add
else
puts "Goodbye!"
end
end
end
add_num = Adder.new()
add_num.class # => Adder
add_num.add
add_num.again
I have the feeling this is supremely simple and I'm just missing it or haven't quite been taught it yet. Any thoughts? Thanks!
2 Answers

Joseph Kato
35,340 PointsYour issue seems to be that you're attempting to reference add_num
from within the class itself, even though add_num
was defined outside of the class definition.
There are many options to fix this issue, but one simple way would be to change (from within the again method):
add_num.add
To just:
add
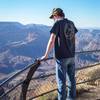
Ricky Catron
13,023 PointsYou could do something like make a while loop and while input not equal to quit run the program else quit the program.
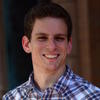
Jeff Lange
8,788 PointsCan you show me a quick example of how I would do that? I tried creating a while loop like this:
def add
while response == "y"
[code]
end
but I got the same error of the variable "response" being undefined, which confused me since, well, near as I can tell I did define it.
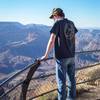
Ricky Catron
13,023 PointsI don't know Ruby sorry. A basic example would be
response = y
while response == y
[code]
[input new variable for response if you want to keep going]
end
Jeff Lange
8,788 PointsJeff Lange
8,788 Pointsahhh!!! You're so right! For some reason I was trying to call the method as if I was outside the class even though i was within it.
In the "again" method I changed it to
add
and in the add method I inserted a call to the again method by just puttingagain
. Now it works exactly like I wanted it to: every single time it adds the numbers it asks if you want to add anymore (y/n), and loops if you type y, and ends if you type n. Awesome!Thank you Joseph! Super helpful! As simple as that was, that should actually solve a lot of issues I've been running into the past few days. You helped me break through a mental barrier.