Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial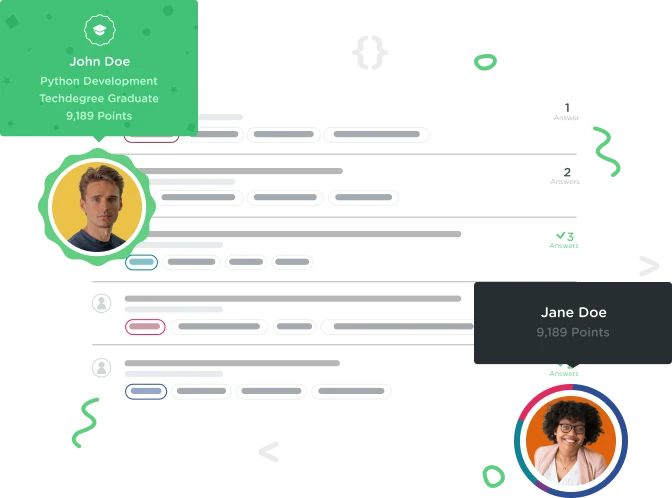
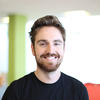
Kristian Woods
23,414 PointsHow do you check if an href contains a certain combination of characters?
I have to check if an href contains a certain combination of characters, and if so - hide that link (<a> tag)
<div id="parent-container">
<a href="#K1">link</a>
<a href="#K1AB">link</a>
<a href="#K1CD">link</a>
<a href="#D2">link</a>
<a href="#D2AB">link</a>
<a href="#K1HHH">link</a>
<a href="#">link</a>
</div>
For the list of codes, I created an array:
let arrayofcodes = [
'K1',
'K1AB',
'K1CD',
'D2',
'D2AB'
];
I then use JQuery to check the a links for the above codes:
$('#parent-container a').each(function () {
let buttonLink = $(this).attr('href').toString();
for (let i = 0; i < arrayofcodes.length; i++) {
if (buttonLink.includes(arrayofcodes[i])) {
$(this).css('background-color', 'blue');
}
}
});
So, the issue I'm having - links that have a code that isn't listed in the array are still being changed to the colour blue. Thats due to the logic of the if statement - its checking to see if each link has an instance of "K1" - and because most links have a code, thats why they are changing colour. I only want to change colours of the links that have the full code and are listed within the array. How can I change/edit the logic?
I've tried using indexOf method - but I run into the same issue
Thanks

KRIS NIKOLAISEN
54,967 PointsThe condition in his code also includes <a href="#K1HHH">link</a> even though 'K1HHH' is not in arrayofcodes. But K1 is.
2 Answers

KRIS NIKOLAISEN
54,967 PointsThis comes up with the results but may not be what you are looking for:
$('#parent-container a').each(function () {
let buttonLink = $(this).attr('href').toString();
for (let i = 0; i < arrayofcodes.length; i++) {
if (buttonLink == "#" + arrayofcodes[i]) {
$(this).css('background-color', 'blue');
$(this).hide();
}
}
});
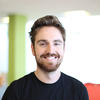
Kristian Woods
23,414 PointsHey, I changed the codes slightly, however the principle is the same.
I figured it out - basically, what I did was add an "&" at the end of the if statement check. As each code ends with an "&"
K1G&, K1&, K1RV&
This way, even if a link has an instance of "K1" - it has to be directly followed by an "&", otherwise it wont pass the check. I also switched the check, and used the indexOf method.
let arrayofcodes = [
'K1G',
'K1',
'K1RV',
'D2RV',
'D2G',
'K1AC'
];
$('#parent-container a').each(function () {
let buttonLink = $(this).attr('href').toString();
for (let i = 0; i < arrayofcodes.length; i++) {
if ($(this).attr("href").indexOf(arrayofcodes[i] + "&") != -1 ) { // ADD "&"
$(this).css('background-color', 'blue');
}
}
});
Thanks for your help, though!
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsI don't think you have any issues with your code - the links are blue because that is the default color. Is that all you were concerned about?