Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial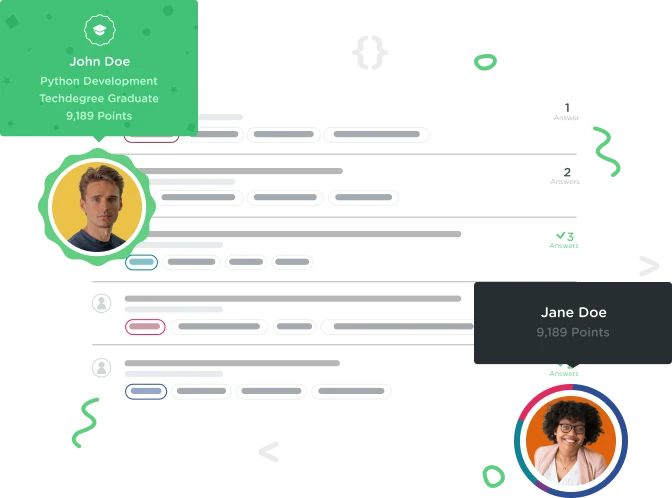
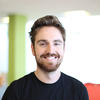
Kristian Woods
23,414 PointsHow do you check the value of a form field?
Hey, I'm trying to create a script that asks users to select a musician and how many tickets they'd like to the gig. Tickets are £10 each, and an additional £5 for each ticket after 4 tickets have been purchased.
I'm having trouble obtaining the value the user selects from the select menu, though.
Any help would be greatly appreciated
Thanks
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tickets</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Nunito:400,300' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<form action="index.html" method="post">
<fieldset>
<legend><span class="number">2</span>Buy Tickets</legend>
<label for="artists">Artists</label>
<select id="artists" name="artists">
<optgroup label="Choose Artist">
<option value="Musician 1">Musician 1</option>
<option value="Musician 2">Musician 2</option>
<option value="Musician 3">Musician 3</option>
<option value="Musician 4">Musician 4</option>
</select>
<label for="job">How Many Tickets?</label>
<select id="job" name="user_job">
<optgroup label="Choose Artist">
<option id="one" value="1">1</option>
<option id="two" value="2">2</option>
<option id="three" value="3">3</option>
<option id="four" value="4">4</option>
<option id="five" value="5">5</option>
<option id="six" value="6">6</option>
</optgroup>
</select>
</fieldset>
<div id="totalPriceDiv">
</div>
<button id="buy" type="submit" onclick="return purchaseFinished();">Buy Tickets</button>
</form>
<script src="ticketPrice"></script>
<script src="validate.js"></script>
</body>
</html>
function displayPrice(price) { // places content within a div
var totalPriceDiv = document.getElementById("totalPriceDiv");
totalPriceDiv.innerHTML = price;
var total = 0;
var finalPrice = " ";
if (document.getElementById("one").value == "1") {
total += 10;
} else if (document.getElementById("two").value == "2") {
total += 20;
}
finalPrice += "<P>Total Price: " + "£" + total + "</p>";
displayPrice(finalPrice);
}
1 Answer
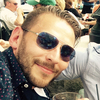
Brian Steele
23,060 Pointsvar foo = document.getElementById('artists');
var bar = foo.value;
When you run that JS it should give you the value you're hoping for. For each corresponding select, you need to grab the select element and check the value. The key is to check the value at the time the user has take a 'submitting action' (whenever that may be for your form)