Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial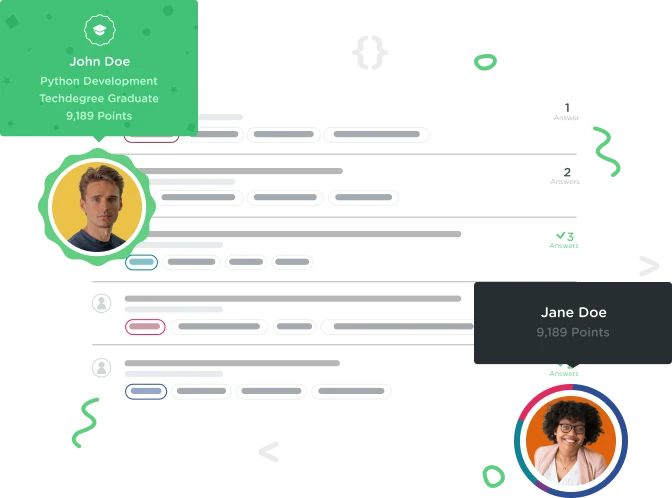

Benjamin Peters
552 PointsHow do you convert arguments to floats before returning them?
See the example for the code that I am trying to work through—I can't convert the arguments to floats before returning them. I've Googled around and can't seem to find an answer that makes sense to me, a super noob.
def add(5, 3):
float(5, 3)
return(5 + 3)
2 Answers
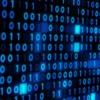
Alexander Davison
65,469 PointsGod job Benjamin! There is a couple exceptions, though. Firstly, for names (variable names, function names, etc.) there are a couple rules:
- Names can't start with a number, but the other characters can
- They also cannot contain a special character such as the question mark, period, or comma
- They can't have spaces, but they CAN have underscores ( _ )
This means these names are valid:
- hello
- var1
- hello_and_bye
And these are invalid:
- 1hello
- right?
- hello and bye
For your second problem, don't get confused when the challenge says "Make your numbers float before adding them together", this means that you should do t WHILE you are calculating. To complete the task, try this code snippet:
def add(a, b):
return float(a) + float(b)
Hope that helps!

Idan Melamed
16,285 PointsHi Benjamin, Being super noob is awesome... It sounds much better then a regular noob!
To turn an argument (which is a variable that's has been passed to a function) to float you call the float() function, which takes an argument and returns it as a float. You then need to assign what the float() function returns back to the argument. Like this:
arg = float(arg)
So for that challenge you either do:
def add(arg1, arg2):
arg1 = float(arg1)
arg2 = float(arg2)
return arg1 + arg2
Or you can do the shorthand version like this:
def add(arg1, arg2):
return float(arg1) + float(arg2)

Benjamin Peters
552 PointsThank you so much! This was really helpful.
Benjamin Peters
552 PointsBenjamin Peters
552 PointsDefinitely helps. I really appreciate it. I'll run it and see what happens. Thanks!