Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial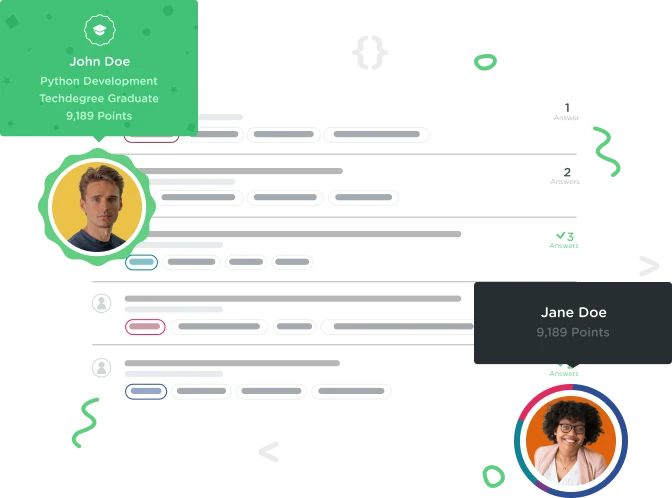

Austin Yeater
5,510 Pointshow do you Convert the greet function declaration to an arrow function. HINT: Arrow functions are not hoisted – or lif
const greet('cool coders') => ;
function greet(val = 'Austin') {
return `Hi, ${val}!`;
}
1 Answer
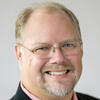
Jason Larson
8,361 PointsYou were on the right track by making the arrow function at the top, but you were working on the wrong part. That first line is calling the function, and since you are converting the function to an arrow function, you leave that line as it is, but move it down below the function declaration. As for the arrow function itself, you are going to remove the 'function' keyword and replace it with let or const, then put an equal sign after the name, then stick the arrow between the parameters and the curly braces:
const greet = (val) => {
return `Hi, ${val}!`;
}
greet('cool coders');
Note that because this is just a single line of code in the function, and it's the return statement, you can shorten this by eliminating the curly braces and the return keyword. (If you have more than one line of code, you'll need to use the curly braces and remember to return
anything you need to get from your function.) You can also get rid of the parentheses around the parameter, though you'll need to have it if you want to use a default parameter like you are trying to do in your code:
const greet = val => `Hi, ${val}!`;
greet('cool coders');
const greet = (val = 'Austin') => `Hi, ${val}!`;
greet();