Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial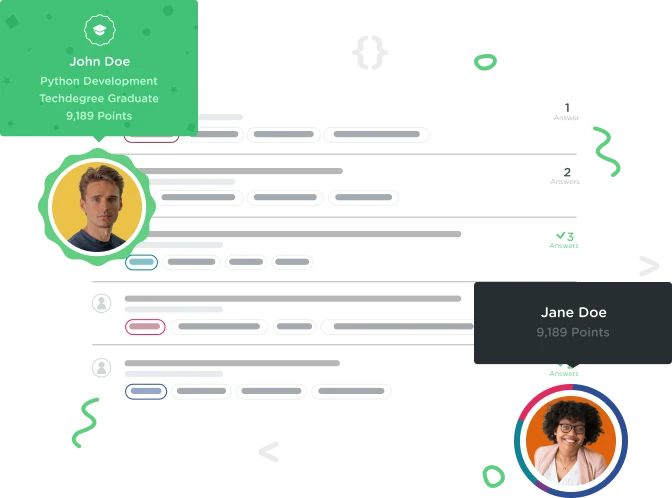

Drew Scolaro
Courses Plus Student 855 PointsHow do you create a dictionary?
Help me by explaining how to make a dictionary
// Enter your code below
var iceCream = ["CC" "AP" "PB"]
4 Answers
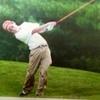
kjvswift93
13,515 PointsDictionaries store unordered list of values of the same type. A dictionary uses a key as an identifier for a value which can then be retrieved later by using the key. Using the code challenge as an example, for the dictionary named iceCream, "CC" is the key which references the string value "Chocolate Chip". If I wanted to retrieve the value "Chocolate Chip" later, I would use it's key "CC" like thus: iceCream["CC"]. In the case of the complete code challenge solution, this would print "Chocolate Chip Cookie Dough" in the results view, seeing as the fourth part of the challenge asks you to change, or update, the value that the key "CC" refers to from "Chocolate Chip" to "Chocolate Chip Cookie Dough". The complete solution to all four parts of the code challenge are below.
var iceCream = ["CC": "Chocolate Chip", "AP": "Apple Pie", "PB": "Peanut Butter"]
iceCream.updateValue("Rocky Road", forKey: "RR")
let applePie = iceCream["AP"]
iceCream.updateValue("Chocolate Chip Cookie Dough", forKey: "CC")

Oliwer Bendelin
6,390 PointsYou have colons after your keys and before your value, then you separate everything with commas

Alexandre Paradis
Courses Plus Student 2,291 Pointsvar airports: [String: String] = ["YYZ": "Toronto Pearson", "DUB": "Dublin"]

Jorge Solana
6,064 PointsSup Drew!
This might be a good example for a dictionary definition and easy use.
let nbaDictionary: [String: String] = ["GSW": "Golden State Warriors",
"LAC": "Los Angeles Clippers",
"CLE": "Cleveland Cavaliers",
"NOP": "New Orleans Pelicans"]
let myTeam: String = "CLE"
let myTeamName = nbaDictionary[myTeam]
print(myTeamName!)
In this example, I write a dictionary of some nba teams for example. Then I tell the program that my favorite team is the one called "CLE", so I get myTeamName from that dictionary and then I print it to see the results. The myTeamName constant will be "Cleveland Cavaliers" (Note).
Note: forget about the ! for the moment, that's an optional and you'll get there soon.