Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial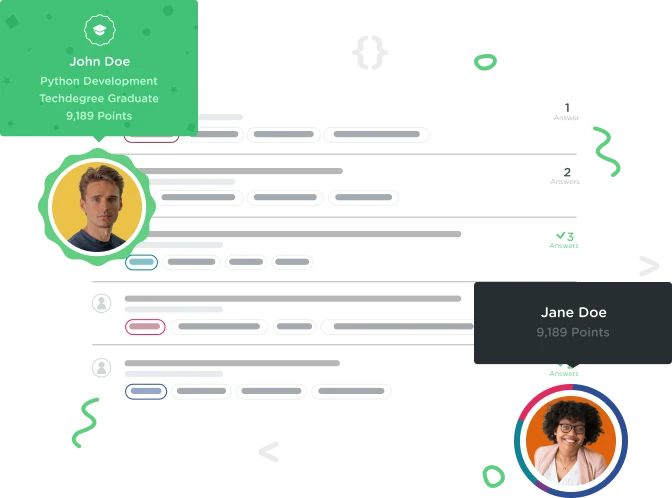
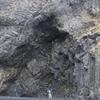
Jay Bhatt
6,502 PointsHow do you create an if statement where I can account for no value being entered for the quantity?
I am attempting to allow the program to take the default quantity to be 1 if the user just entered addItem(dispenser) and not addItem(dispenser,1). I have been stuck on this for the past 2 days. Please help!
Thank you!
P.S I know my code is wrong, I just don't know how to design the if statement to account for the no number entered.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
// cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
if (int quantity <= 0) {
int quantity =1
public void addItem(Product item, int quantity){
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
}
}
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
1 Answer

andren
28,558 PointsWhile I can kind of see what you are trying to do, that type of code will never work due to the way Java works, when you call a method java doesn't just look at the name of the method, it looks at the name and the amount & type of arguments provided and then looks for methods with both a matching name and a matching number & type of parameters.
In other words when addItem is called with only one argument Java will look for a method called addItem that only takes one argument of whatever type you supplied it with, if it cannot find it Java will not default to the addItem method that takes two arguments like you assume, but will instead simply crash.
The way to solve this issue is to create a new method called addItem that does only take one parameter, and then make a call within this method to the other addItem method where you hardcode the second argument.
Here is some example code that shows the solution:
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
public void addItem(Product item) {
addItem(item,1);
}
}
if you still have questions about this concept I would recommend that you lookup the term "Method Overloading" as that should clarify some of your confusion.
Edit: Added some clarification and fixed some typos.
Jay Bhatt
6,502 PointsJay Bhatt
6,502 PointsThat is beautifully simplistic. Thank you so much. It just clicked on how easy that was.