Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial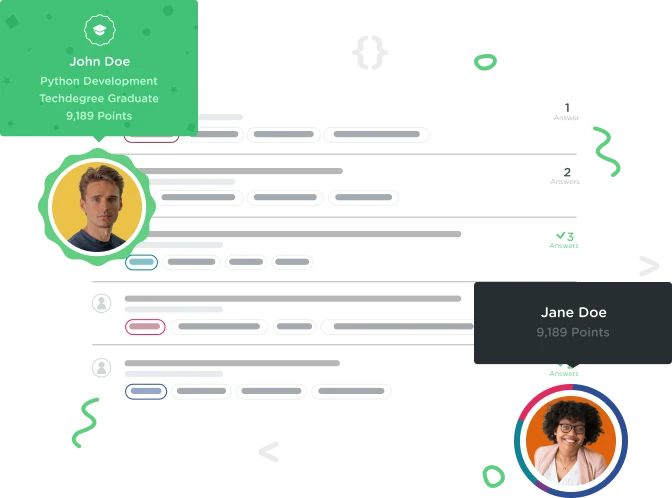

alex santorineos
4,345 Pointshow do you do the extra credit for stage 5 in the self destructing messaging app for iOS? Any ideas?
Please help
3 Answers

Ben Jakuben
Treehouse TeacherThis is a tough one! You need to construct a URL request, configure it, and then send it to Parse. The best example I've found so far is an answer on StackOverflow: http://stackoverflow.com/a/25020615/475217
Give that a shot and let us know if you have any follow-up questions. You'll have to watch the backend in your Parse dashboard to verify the delete. Good luck!

alex santorineos
4,345 Points#import "inboxViewControler.h"
#import "ImageViewController.h"
@interface inboxViewControler ()
@end
@implementation inboxViewControler
- (void)viewDidLoad {
[super viewDidLoad];
self.moviePlayer = [[MPMoviePlayerController alloc] init];
PFUser *currentUser = [PFUser currentUser]; //show current user in console
if (currentUser) {
NSLog(@"Current user: %@", currentUser.username);
}
else {
[self performSegueWithIdentifier:@"showLogin" sender:self];
}
}
-(void)viewWillAppear:(BOOL)animated{
[super viewWillAppear:animated];
PFQuery *query = [PFQuery queryWithClassName:@"Messages"];
// [query whereKey:@"recipientIds" equalTo:[[PFUser currentUser] objectId]];
[query orderByDescending:@"createdAt"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error: %@ %@", error, [error userInfo]);
}
else {
// We found messages!
self.messages = objects;
[self.tableView reloadData];
NSLog(@"Retrieved %d messages", [self.messages count]);
}
}];
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
// Return the number of rows in the section.
return [self.messages count];
return 0;
}
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
PFObject *message = [self.messages objectAtIndex:indexPath.row];
cell.textLabel.text = [message objectForKey:@"senderName"];
NSString *fileType = [message objectForKey:@"fileType"];
if ([fileType isEqualToString:@"image"]) {
cell.imageView.image = [UIImage imageNamed:@"icon_image"];
}
else {
cell.imageView.image = [UIImage imageNamed:@"icon_video"];
}
return cell;
}
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath{
self.selectedMessage = [self.messages objectAtIndex:indexPath.row];
NSString *fileType = [self.selectedMessage objectForKey:@"fileType"];
if ([fileType isEqualToString:@"image"]) {
[self performSegueWithIdentifier:@"showImage" sender:self];
}
else {
// File type is video
PFFile *videoFile = [self.selectedMessage objectForKey:@"file"];
NSURL *fileUrl = [NSURL URLWithString:videoFile.url];
self.moviePlayer.contentURL = fileUrl;
[self.moviePlayer prepareToPlay];
// [self.moviePlayer thumbnailImageAtTime:0 timeOption:MPMovieTimeOptionNearestKeyFrame];
// Add it to the view controller so we can see it
[self.view addSubview:self.moviePlayer.view];
[self.moviePlayer setFullscreen:YES animated:YES];
}
//delete it
// Delete it!
NSMutableArray *recipientIds = [NSMutableArray arrayWithArray:[self.selectedMessage objectForKey:@"recipientIds"]];
NSLog(@"Recipients: %@", recipientIds);
if ([recipientIds count] == 1) {
// Last recipient - delete!
[self.selectedMessage deleteInBackground];
}
else {
// Remove the recipient and save
[recipientIds removeObject:[[PFUser currentUser] objectId]];
[self.selectedMessage setObject:recipientIds forKey:@"recipientIds"];
[self.selectedMessage saveInBackground];
//[self.deleteMessages delete:fileType]; - - - TRY TO DELETE FILE
[recipientIds removeObject:[[PFUser currentUser] objectId]];
[self.selectedMessage setObject:recipientIds forKey:@"recipientIds"];
[self.selectedMessage saveInBackground];
}
}
- (IBAction)logOut:(id)sender {
[PFUser logOut];
[self performSegueWithIdentifier:@"showLogin" sender:self];//logout of account
}
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([segue.identifier isEqualToString:@"showLogin"]) {
[segue.destinationViewController setHidesBottomBarWhenPushed:YES];
}
else if ([segue.identifier isEqualToString:@"showImage"]) {
[segue.destinationViewController setHidesBottomBarWhenPushed:YES];
ImageViewController *imageViewController = (ImageViewController *)segue.destinationViewController;
imageViewController.message = self.selectedMessage;
}
}
@end
```
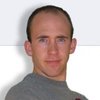
Trevor Duersch
9,964 PointsAlex, all you need to do is follow Ben's suggestions (i.e. From this website: http://stackoverflow.com/a/25020615/475217).
I found it was a lot easier to follow and it wasn't as complex as I thought it would be. I was thinking I would have to create a JSON object to send in the request body but it turns out that I didn't need to actually.
I hope that helps!
alex santorineos
4,345 Pointsalex santorineos
4,345 PointsDo I need to create a whole new class for this?
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherNo, but I would recommend creating a new method. In your current code that deletes the message, call a new method. Name it something like
deleteFileFromBackend
and then add this code (with a few modifications) to that new method.alex santorineos
4,345 Pointsalex santorineos
4,345 Pointscan you help me/assist me with the method. What should the type be? Should it be -(void) deleteFileFromBackend; or should there be more to it?
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherYep - void is correct! It's just a helper method that will allow your code to be more organized.
alex santorineos
4,345 Pointsalex santorineos
4,345 Pointsdoes there need to be a parameter for that method?
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherIt totally depends on how your current code is, but probably. I imagine you'll want to pass in the ID of the file you are trying to delete, or maybe even a PFFile if you already have a variable holding it.
alex santorineos
4,345 Pointsalex santorineos
4,345 Pointscan you please guide me through that. I don't quite understand the last thing you said.
alex santorineos
4,345 Pointsalex santorineos
4,345 PointsI am still very new to this. I have been doing this for only two months. It would be nice if somehow there was step by step guidance.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherLet's do this - show me the code you are trying to write and we can talk about it from there. We don't give much support to extra credit because the intent is to complete it as an extra challenge without much teacher input. But using the Forum like this is a great way to try to work through issues, and it helps to talk in details about the code.