Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial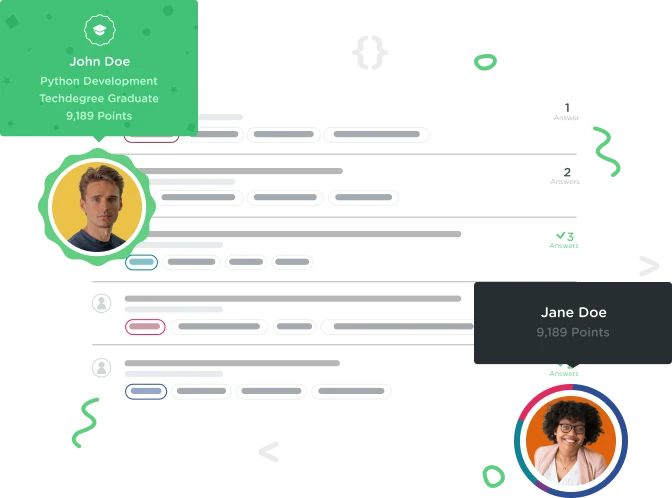

homer simpson
281 Pointshow do you do this
ists hard
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers[counter])
counter += 1
}
sum += counter
2 Answers

Dominic Gallo
2,774 PointsThe problem is asking you to sum all the values in the array with a while loop. It gives you some initial values and sets you up wit the variables they want you to use. First you have to think about how you're going to solve the problem in your head. The way I did it was, I wanted to start adding everything in the array starting in the first spot, numbers[0] and add all the way up until we got to the end of the array [numbers.count]. One way you can do it in swift is,
while counter < numbers.count
Since they set counter = 0
for you already, then this should read that while 0 is less than the whole length of the array do this
{
sum = sum + numbers[counter]
counter += 1
}
since sum = 0, then the body of the while loop on the first iteration says, 0 = 0 + whatever is in the first spot of the array
next step is to increase the counter so we move onto the next spot in the array
then the second iteration is, whatever was in the first spot of the array = whatever was in the first spot of the array + the next spot
this goes on until everything is added. Donezo
Here is a working example as of swift 4.1 on 14 APR 18
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum = sum + numbers[counter]
counter += 1
}
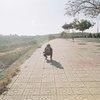
Johnny Nguyen
3,875 Pointswe use "counter" to track the number of iterations of the while loop. So we increase counter until it's less than "numbers.count"
while counter < numbers.count {
sum += numbers[counter] //sum = sum + numbers[counter]
counter += 1
}