Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial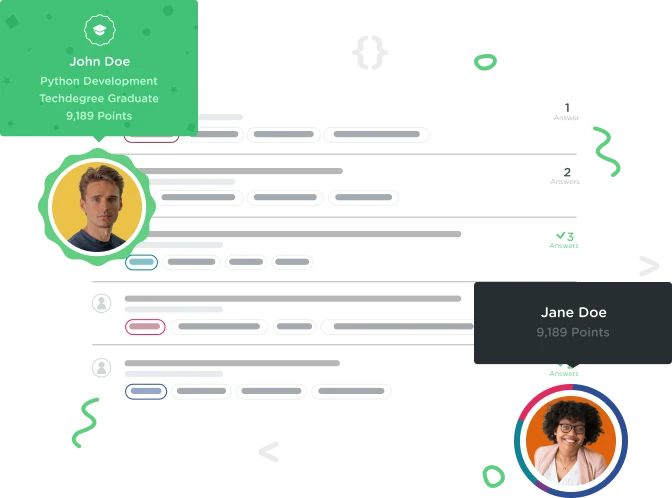
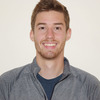
Connor Croteau
1,160 PointsHow do you do this question? I don't understand how to incorporate the temperatures array correctly.
What am I doing wrong here?
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 0; i < temperatures.length; i += 1) {
console.log(i);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
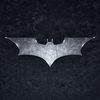
Logan R
22,989 PointsYou're mostly there!
To get the value from an array, you must first do the name of the array, followed by the offset in [].
So for example:
var myArray = [10, 40, 50]
So say that I wanted the 40 from the array. I would get this by using an offset of 1 (Starting from 0):
console.log(myArray[1]);
So in this instance, you want an offset of i.
Does that make sense?
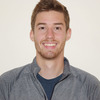
Connor Croteau
1,160 PointsHmmm but how do you log the array out in order then? Whats the syntax for that?
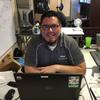
Amos Dailey
18,885 PointsInside your loop you will log the array out using the var "i" as the counter.
So the first time through the loop, "i" will be 0. then it hits console.log(temperature[i]); ///Which is the same as console.log(temperature[0]);
And on the next iteration through the loop "i" will be one. So... console.log(temperature[i]); ///Which is the same as console.log(temperature[1]);
And so on till you get to the length of the array.