Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial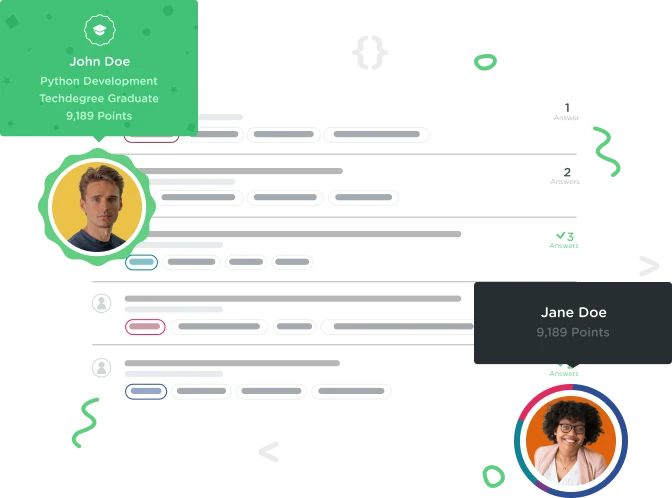

MUZ140847 Ralph Dendere
3,690 Pointshow do you get a function to take values from a list
def add_list(list1): for number in list1: print(number) sum_of_list = number+number return sum_of_list
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
def add_list(list1):
for number in list1:
sum_of_list = number+number
return sum_of_list
1 Answer

Chris Adamson
132,143 PointsIn your code example, you have effectively created a sum_of_list variable scoped to the for loop. Also the return statement is in the for loop. You need to declare the sum_of_list variable before the start of the for loop, then in the for loop keep adding number to sum_of_list, and then return sum_of_list outside of the loop:
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
def add_list(list1):
sum_of_list = 0
for number in list1:
sum_of_list += number
return sum_of_list
MUZ140847 Ralph Dendere
3,690 PointsMUZ140847 Ralph Dendere
3,690 Pointsthank you