Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial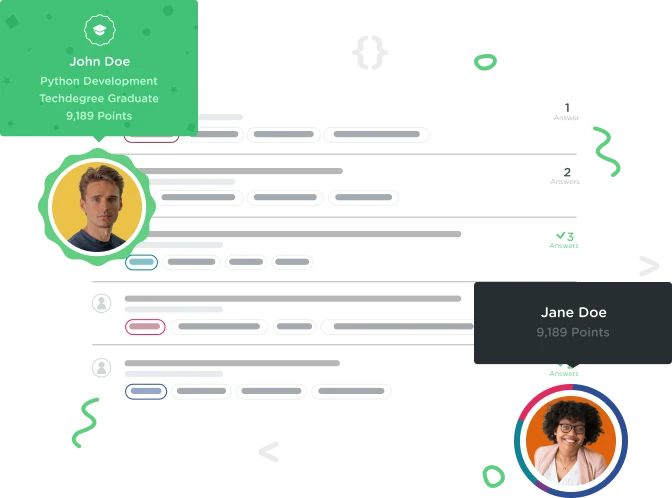

Martin Opheim
789 PointsHow do you know what interface to implement, and why would you?
How do you know what interface to implement and why would you implement an interface if it for example would make you have to implement a method that was not necessary for what you are making?
Also, are interfacing policed in companies by having a set of coding rules and having code review? Or there any other ways to make sure someone implements an interface?
3 Answers
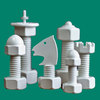
Steven Parker
229,783 PointsYou'd typically implement an interface to make it possible to do certain things with your object. For example, to be able to use sorting functions, your object needs to implement IComparable
. Or to make an object easily displayed it would implement IFormattable
.
Interfaces often require you to implement only the minimum features needed to do the task. For example, both of those I mentioned previously contain only one method. A well-designed interface is not likely to require you to do things you won't need.
Coding rules vary widely among companies, but generally the specification for your code will indicate which interfaces you should use. Attempting to meet the specification without using the interface would generally be far more complicated and not worth the effort.

Lee Owen
7,907 PointsThat makes sense, thanks Steven.

Carel Du Plessis
Courses Plus Student 16,356 PointsI don't quite understand how implementing an interface will help you do certain things with your object and how it effects the DRY practice. when is it better to use (virtual and override) or abstract.
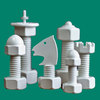
Steven Parker
229,783 PointsThe example in my original answer would be a good case for implmenting the IComparable
interface. If you wanted to be able to use the standard sorting functions, your object needs to implement that one for them to work.
The functions in the interface are abstract, when you implement the interface you provide the actual code.
Lee Owen
7,907 PointsLee Owen
7,907 PointsHey Steven,
Branching off on this, would it be good practice to make an interface for every object, and every method that it has?
Say you're making an app that tracks information about different race cars. So you have a base RaceCar class that defines what it means to be a race car and all of its functions, but then interfaces that just define the functions by making something like...
interface ITires interface IEngine interface ITransmission interface IRaceCar : ITransmission, IEngine, ITires
then my abstract RaceCar class would inherit the IRaceCar interface, and implement whatever methods it needed, and then a subclass of my RaceCar, say IndyCar would inherit from RaceCar by IndyCar : RaceCar, or would I just skip all of this and just inherit from the interface and skip the RaceCar base class?
Any clarification would be appreciated!
Steven Parker
229,783 PointsSteven Parker
229,783 PointsHaving "IndyCar" inherit from "RaceCar" makes sense, but breaking down "RaceCar" into multiple interfaces is probably not worthwhile unless each interface would be likely to be used in a number of other unrelated classes also.