Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial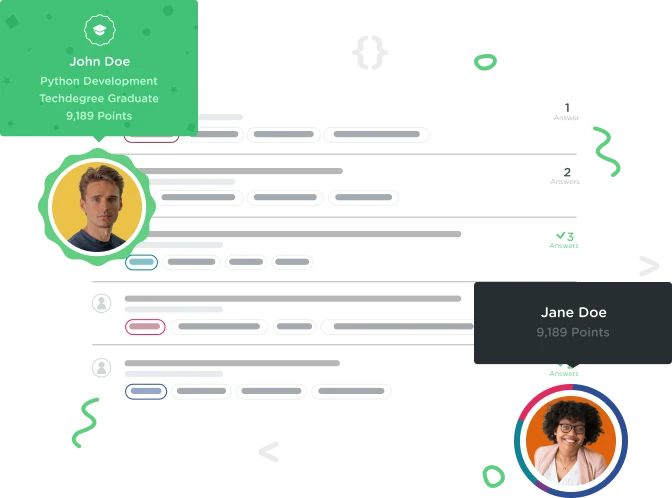

Andrea Fung
Courses Plus Student 4,713 PointsHow do you output an instance of a class as a string?
To practice OOP, I tried to create an an item class and inventory class. My inventory contains a list of items (self.in_stock). When I print out my list, I get the location of each item in memory. I have created a str method for class, so why do I not see a list of strings?
I have copied my code below:
class Item:
def __init__(self, item_name, brand, colour, build_cost):
self.item = item_name
self.colour = colour
self.brand = brand
self.sold_out = False
self.build_cost = build_cost
self.price = build_cost * 10
def __str__(self):
return f'{self.colour} {self.item} by {self.brand}'
def __hash__(self):
return hash((self.item, self.colour, self.brand, self.sold_out, self.build_cost, self.price))
def __eq__(self, other):
return (self.item == other.item) and (self.colour == other.colour) and (self.brand == other.brand)
class Inventory:
def __init__(self):
self.in_stock = []
self.sold = []
self.brands = set()
self.columns = ['Item', 'Price', 'Quantity in stock', 'Quantity sold']
def __iter__(self):
yield from self.in_stock
def add_item(self, item, quantity):
new_item = item
self.in_stock.extend([new_item] * quantity)
[MOD: added ```python formatting -cf]
2 Answers
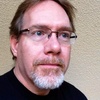
Chris Freeman
Treehouse Moderator 68,423 PointsHey Andrea Fung, it might be helpful to see the code you used to create the instances and how your are printing.
If you are printing the in_stock
attribute, the attribute is a list
, therefore it is the list.__str__
method being used to print it.
What you may want is a method in Inventory that prints in_stock
such as
def print_stock(self):
return “”.join([str(item) for item in self.in_stock])
results in:
i = Inventory()
i.add_item(Item('item1', 'brand1', 'color1', 1), 1)
i.add_item(Item('item2', 'brand2', 'color2', 2), 2)
print(i)
print(i.in_stock[0])
print(i.print_stock())
#output
<__main__.Inventory object at 0x106a8ef60>
color1 item1 by brand1
color1 item1 by brand1, color2 item2 by brand2, color2 item2 by brand2
If this is to be the default string interpretation of an Inventory
, the above method could be renamed to __str__
Post back if you need more help. Good luck!!!

Andrea Fung
Courses Plus Student 4,713 PointsThank you very much for the clarity, Chris! I have been going through your responses for other questions from the community and it has been so helpful for my learning. Thank you!