Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial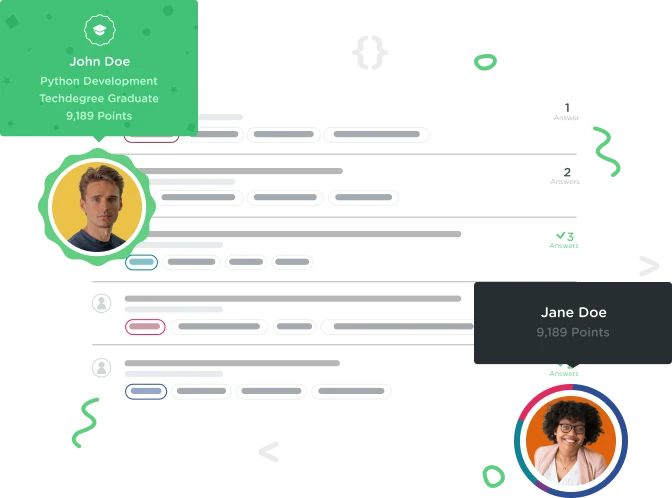
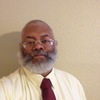
Adiv Abramson
6,919 PointsHow do you prevent an object from being instantiated if the call is syntactically valid?
For example, if we have a constructor function in Javascript (which to me seems to be the equivalent of a class definition in Java), how can we make the invocation fail intentionally if one or more parameters falls outside an accepted domain? Thanks!
function myObject(height, length, width) {
if (isNaN(height) || isNaN(length) || isNaN(width)) {
/*what do I write here so that a new instance of myObject
is NOT created? JS doesn't isn't strongly typed so an NaN
error would not be caught at compile time */
}// if (isNaN(height) || isNaN(length) || isNaN(width))
}//myObject
var myBadObject = new myObject("rtvc", false, Math.sqrt(2));
Perhaps this is a case where a constructor function should return a value to indicate success or failure?
3 Answers

Rich Donnellan
Treehouse Moderator 27,671 PointsAdiv,
Building off of Justin's answer, you'd be better suited using the typeof
operator due to isNaN
's ability to throw false positives. The AND (&&)
logical operator must be using instead of OR (||)
, as it will return true
if any case is true
(not what we want here).
Notice the NOT (!)
operator on the grouped if
:
function Object(height, length, width) {
if (!(typeof height === 'number' && typeof length === 'number' && typeof width === 'number')) {
throw new Error("All parameters must be numbers.");
} else {
this.height = height;
this.length = length;
this.width = width;
console.log("Congrats! These are all numbers.");
}
}
//Test data
var badObject = new Object('rtvc', false, Math.sqrt(2));
//var goodObject = new Object(6, 12, Math.sqrt(2));
Hope this helps some.
– Rich

Justin Perry
12,530 PointsAdiv, you can throw an exception to stop the instantiation of the object.
function Object(height, length, width) {
if (isNaN(height) || isNaN(length) || isNaN(width)) {
throw new Error(" parameters must be numbers");
} else {
this.height = height;
this.length = length
this.width = width;
}//myObject
}
var myBadObject = new Object("rtvc", false, Math.sqrt(2));
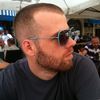
Matija Komorčec
13,123 PointsRich, we can use OR operator too, because it doesn't matter if we enter 1 parameter wrong, or all 3, we still want to throw an error. But the way you grouped the IF would work great.

Iain Simmons
Treehouse Moderator 32,305 PointsI'm trying to follow that logic in my head... if you use OR, then you need a !
before each part. Otherwise if you used OR within a group that has the !
on the outside, it would stop at the first true statement on the inside...
...right?