Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial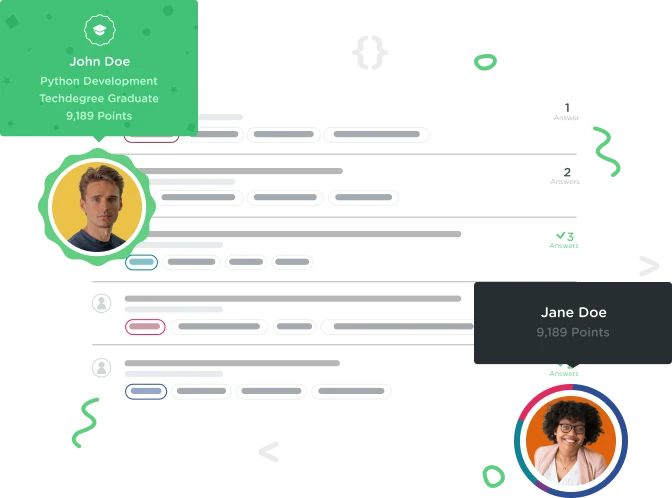
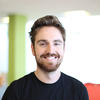
Kristian Woods
23,414 PointsHow do you remove duplicate items from an Set in Javascript?
I'm trying to generate 52 playing cards at random.
I have a constructor that create a newCard object. Each object is assigned a random number between 2-10 and a suit.
I then use a While loop to generate the newCard object 52 times. However, I'm generating duplicate cards. How can I resolve this? I tried using a javascript Set() opposed to an array, because I thought it wouldn't duplicate the cards.
Also, it would be really apprecatied it someone could comment on my code, and suggest an alternative to refactor the code.
Thanks
Code below:
Constructor function:
class PlayingCard {
constructor(number, suit) {
this.suit = suit;
this.number = number;
}
get cardInfo() {
return `The Card is the ${this.number} of ${this.suit}`;
}
}
Random Number(rN) generator (2-10):
let ranNumber = () => {
let rN = Math.floor(Math.random() * 10) + 1;
if(rN == 1) {
rN += 1;
} else if(rN == 11) {
rN -= 1;
}
return rN;
};
Suit Array and While loop:
const suitArray = [
'Hearts',
'Diamonds',
'Clubs',
'Spades'
];
let randomSuit = () => suitArray[Math.floor(Math.random()*suitArray.length)];
let cardSet = new Set();
let i = 52;
while(i) {
let newCard = new PlayingCard(ranNumber(), randomSuit());
cardSet.add(newCard);
paragraph(newCard.cardInfo);
i--;
}
1 Answer
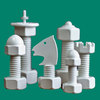
Steven Parker
231,275 PointsSets require unique entries, but the test for equality on an object is actually a test for identity. Two different objects with the same properties are still considered not equal.
You could use a loop to remove duplicates, but then you "won't be playing with a full deck".
If you really want a deck of cards, why not just generate them in a non-random way?
for (suit of suitArray)
for (number = 1; number <= 13; number++)
cardSet.add(new PlayingCard(number, suit));
Note: I extended the numbers to include 1 (ace) and 11-13 (jack, queen, king).